Logging: What Is It?
The technique of recording events in real time and adding details like execution time and infrastructure information is known as logging in software. It is essential to every software program, especially when debugging problems. Logs are useful for deciphering errors, locating performance snags, and assisting in issue solving.
Logs are commonly kept in files, databases, or consoles according to the convenience and severity of the application. Although logs can contain a variety of data, error and informational messages are frequently recorded. Informational messages record routine activities such as method calls, user logins, or product checkouts, whereas error messages offer detailed information for troubleshooting.
By offering a generic logging interface that is uniform throughout the framework and external libraries, ASP.NET Core streamlines logging. Problem diagnosis and log navigation are made easier by this standardization. Users can set log verbosity and route logs to various locations, including files, consoles, and databases, using the framework.
Logs are stored by logging providers in ASP.NET Core, and users can set up several providers for their apps. Logging providers like Console, Debug, EventSource, and EventLog (unique to Windows) are part of the standard ASP.NET Core configuration.
Recognizing the Difference: Software System Logging vs. Monitoring
ASP.NET Core has helpful tools for logging—a process of recording events in your applications. System events, user actions, performance indicators, and other events and processes are all recorded in detail through logging. This data, which is commonly kept in a database or log file, enables you to see problems and resolve them, enhance performance, and comprehend how your program is utilized.
Contrarily, monitoring entails keeping an eye on and gauging the performance of your application in real time. When specific criteria are met, such as when the program takes too long to react or experiences an error, it can send warnings or notifications. Monitoring offers important insights for performance optimization and assists in identifying and resolving issues before they affect users.
There are well-known tools in ASP.NET Core, such as Microsoft.Extensions.Helping with logging include Serilog, NLog, and logging. These tools assist you in maintaining a robust and effective software system by making it simpler to handle and analyze the data that your application generates.
Additional Information
Logging in.NET Core allows you to monitor activity within your application. It aids in behavior monitoring, problem solving, and performance analysis. Logging is made organized and efficient with the help of the ILogger API. You can store logs in several locations using different logging providers; built-in and third-party choices are available.
Let's now discuss the distinctions between tracing and logging. The goal of logging is to capture important events in your program and produce a sort of summary. However, tracing goes beyond by providing you with an in-depth overview of all the actions taking place within your application, providing a comprehensive history of its operations.
The six main logging levels in .NET
- Critical: This is for really serious issues that could make your app stop working, like running out of memory or space on the disk.
- Error: Use this when something goes wrong, like a database error preventing data from being saved. The app can still work for other things despite encountering errors.
- Warning: Not as severe as errors, but it indicates a potential problem that might lead to more serious errors. It's a heads-up for administrators.
- Information: Gives details about what's happening in the app. Helpful for understanding the steps leading to an error.
- Debug: Useful during development for tracking detailed information. It's not typically used in a live/production environment.
- Trace: Similar to Debug but may include sensitive info. Rarely used and not utilized by framework libraries.
Implement the External Logging Source to Logging Information of API and Windows Service - Serilog
Packages need: Serilog.AspNetCore
- Dot Net CLI - dotnet add package Serilog.AspNetCore --version 8.0.0
- Package Manager - Install-Package Serilog.AspNetCore -Version 8.0.0
Confiuration of Serilog in API
Settings File
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*",
"Serilog": {
"Using": [ "Serilog.Sinks.File" ],
"MinimumLevel": {
"Default": "Information",
"Override": {
"Microsoft": "Warning",
"System": "Warning"
}
},
"WriteTo": [
{
"Name": "File",
"Args": {
"path": "C:/Log001/log_.log",
"rollOnFileSizeLimit": true,
"rollingInterval": "Day"
}
}
]
}
}
Application Startup updates for Logging
using Serilog;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
/// Step 1
builder.Host.UseSerilog((context, configuration) =>
{
configuration.ReadFrom.Configuration(context.Configuration);
}
);
var app = builder.Build();
/// Step 2
app.UseSerilogRequestLogging();
app.Run();
Using the setup to Log actions.
In this setup, we employ the "Assembly - Microsoft.Extensions.Logging.Abstractions, Version=8.0.0.0" for logging actions, and we utilize Serilog to write these logs into files. The ILogger interface is injected into the constructor to facilitate logging functionality.
private readonly ILogger<WeatherForecastController> _logger;
public WeatherForecastController(ILogger<WeatherForecastController> logger)
{
_logger = logger;
}
[HttpGet(Name = "GetWeatherForecast")]
public IEnumerable<WeatherForecast> Get()
{
_logger.LogInformation("Method Entered");
var list = Enumerable.Range(1, 5).Select(index => new WeatherForecast
{
Date = DateOnly.FromDateTime(DateTime.Now.AddDays(index)),
TemperatureC = Random.Shared.Next(-20, 55),
Summary = Summaries[Random.Shared.Next(Summaries.Length)]
})
.ToArray();
string message = $"WeatherForecast Count {list.Length}";
_logger.LogInformation(message);
_logger.LogInformation("Method Exit");
return list;
}
Now, to test the logging functionality, run the application and inspect the specified path. Refer to the image below for guidance.
Application Startup updates for Logging
using Serilog;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
/// Step 1
builder.Host.UseSerilog((context, configuration) =>
{
configuration.ReadFrom.Configuration(context.Configuration);
}
);
var app = builder.Build();
/// Step 2
app.UseSerilogRequestLogging();
app.Run();
Using the setup to Log actions.
In this setup, we employ the "Assembly - Microsoft.Extensions.Logging.Abstractions, Version=8.0.0.0" for logging actions, and we utilize Serilog to write these logs into files. The ILogger interface is injected into the constructor to facilitate logging functionality.
private readonly ILogger<WeatherForecastController> _logger;
public WeatherForecastController(ILogger<WeatherForecastController> logger)
{
_logger = logger;
}
[HttpGet(Name = "GetWeatherForecast")]
public IEnumerable<WeatherForecast> Get()
{
_logger.LogInformation("Method Entered");
var list = Enumerable.Range(1, 5).Select(index => new WeatherForecast
{
Date = DateOnly.FromDateTime(DateTime.Now.AddDays(index)),
TemperatureC = Random.Shared.Next(-20, 55),
Summary = Summaries[Random.Shared.Next(Summaries.Length)]
})
.ToArray();
string message = $"WeatherForecast Count {list.Length}";
_logger.LogInformation(message);
_logger.LogInformation("Method Exit");
return list;
}
Now, to test the logging functionality, run the application and inspect the specified path. Refer to the image below for guidance.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
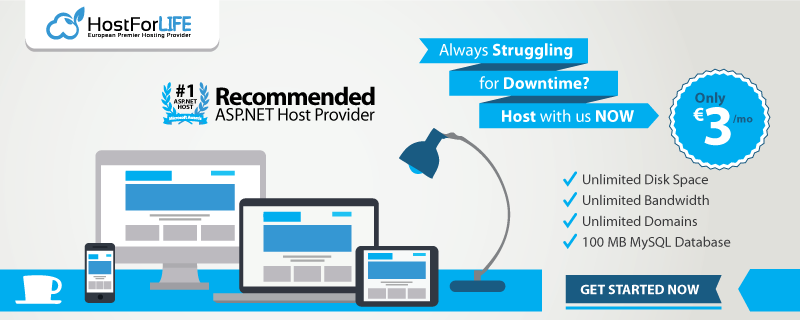