.NET core support build-in dependency injection(DI) which we can achieve by the below approaches:
Constructor injection
Property injection
Method injection
In this article, we will learn
Inject the dependency injection using first method(Constructor Injection)
Register the interfaces and classes in the container class.
To start, few things are required:
Visual studio 22
.NET 6 (.NET Core)
Swagger for testing the API
Before start, I would like to explain few points about the .NET 6 (.NET core new Version 6)
On the creation of the Web API .NET Core project, Startup class is not present.
Startup class gets merged with Program class.
All configurations and services will be configured in the Program class.
Build-in support of Swagger. (Tick the checkbox of "Enable OpenAPI support" while creating the project)
Let's go and start to implement the multiple interface in the .NET 6/.NET Core.
Search and select an ASP .NET Core Web API project from the create a new project tab.
Click next and add the project name
Select .NET 6.0 as framework and click on the check box of "Enable OpenAPI support" as its build-in feature of swagger for testing the API.
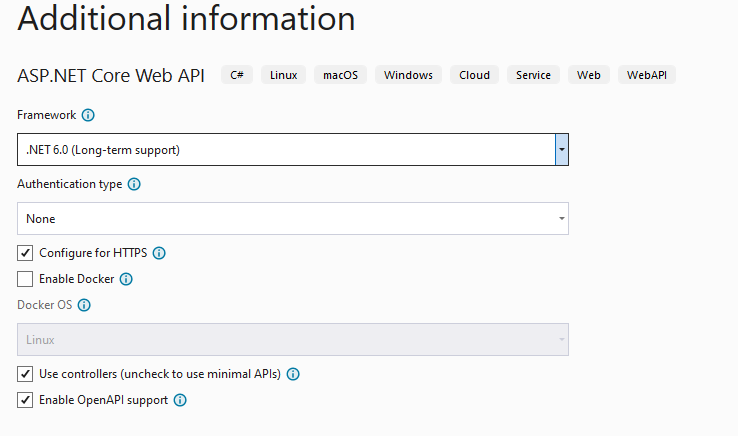
Once the project gets created, then move it to the next step.
STEP 1 - Created interfaces – IEmployeeDetails and IDepartmentDetails
namespace Business;
public interface IEmployeeDetails
{
public List<Employee> GetEmployee();
}
public interface IDepartmentDetails
{
List<Department> getDepartmentDetails();
}
STEP 2 - Create service and implement the interface in the classes as below:
namespace Business;
public class EmployeeService : IEmployeeDetails, IDepartmentDetails
{
public List<Employee> GetEmployee()
{
var employees = new List<Employee>()
{
new Employee()
{
Id = 1,
Title = "Mr",
Name = "Simon",
Age = 32,
EmailId = "[email protected]",
MobileNumber= "12346",
Address = "Pune",
Pincode = 411057
},
new Employee()
{
Id = 2,
Name = "David",
Age = 35,
EmailId = "[email protected]",
MobileNumber= "654323456",
Address = "Mumbai",
Pincode = 221011
},
new Employee()
{
Id = 3,
Title = "Mr",
Name = "Peter",
Age = 29,
EmailId = "[email protected]",
MobileNumber= "54323456",
Address = "Lucknow",
Pincode = 221100
}
};
return employees;
}
public List<Department> getDepartmentDetails()
{
var departmentList = new List<Department>()
{
new Department()
{
DepartmentId = "D001",
DepartmentHead = "Mr. Davis",
DepartmentName = "IT"
}
};
return departmentList;
}
public IEnumerable<Employee> SaveEmpAsList(Employee request)
{
List<Employee> emp = new List<Employee>();
emp.Add(request);
return emp;
}
public Employee GetOneEmployee()
{
Employee employee = new Employee()
{
Id = 3,
Title = "Mr",
Name = "Peter",
Age = 29,
EmailId = "[email protected]",
MobileNumber = "54323456",
Address = "Lucknow",
Pincode = 221100
};
return employee;
}
}
STEP 3 - Need to call the business logic in the controller. For this we need to inject the dependency in the controller layer using Constructor injection
[Route("api/[controller]")]
[ApiController]
public class EmployeeController : ControllerBase
{
private readonly IEmployeeDetails _employeeService;
private readonly IDepartmentDetails _departmentService;
public EmployeeController(IEmployeeDetails employeeService,
IDepartmentDetails departmentService)
{
_employeeService = employeeService;
_departmentService = departmentService;
}
}
STEP 4 - Calling the service using the injector
[Route("GetEmp")]
[HttpGet]
public IEnumerable<Employee> GetEmployeeList1()
{
var res = _employeeService.GetEmployee();
return res;
}
[Route("GetDepartment")]
[HttpGet]
//[Authorize]
public IEnumerable<Department> GetDepartment()
{
var res = _departmentService.getDepartmentDetails();
return res;
}
NOTE: Try to run the code, you will get the run time exception.
"System.InvalidOperationException: Unable to resolve service for type 'Business.IDepartmentDetails' while attempting to activate 'POCAutomapperWithSql.Controllers.EmployeeController'. "
As we haven't registered the interface in the container class (Program.cs). For registering the interface and classes, you need to go in the Program class (As Startup class is no more with .NET 6) and use these methods i.e "AddScoped" || "AddTransient" || "AddSingleton" as it defines the lifetime of the services.
STEP 5 - Go to Program class and register it.
// Register interface and class which we injected
// Register interface and classes
builder.Services.AddScoped<IEmployeeDetails, EmployeeService>();
builder.Services.AddScoped<IDepartmentDetails, EmployeeService>();
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
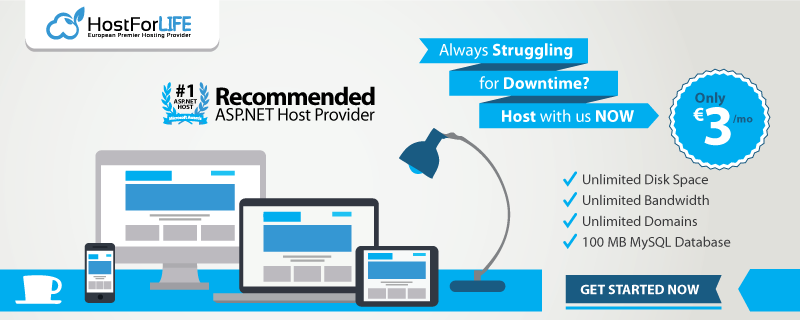