
December 17, 2019 11:29 by
Peter
In web applications, security is essential. Say that the user wants to use the resources of our system. For that, we need to authenticate the user. Authentication means need to check whether the user is eligible to use the system or not. Generally, we do authentication via username (in the form of a unique user name or email ID) and a password. If the user is authenticated successfully, then we allow that user to use the resources of our system. But what about subsequent requests? If the user has been already identified then s/he does not need to provide credentials each time. Once authenticated, for a particular period s/he can use the system's resources. In a traditional approach, we used to save Username in Session. This session period is configurable, which means the session is valid for about 15 or 20 minutes. This session is stored in server's memory. After expiration of the session, the user needs to login again.
But here, there are couple of problems.
- The session can be hijacked.
- If we have multiple instances of server with load balancer, then if the request goes to a server other than the server which has authenticated the earlier request, then it will invalidate that session. Because the session is not distributed among all the servers, we have to use a 'Sticky' session; that is we need to send each subsequent request to the same server only. Here, we can also store session in database instead of the server's memory. In that case, we need to query the database each time, and that's extra work which may increase the overall latency.
To solve this problem, we can do authentication via JWT i.e. JSON web token. After successful authentication, the server will generate a security token and send it back to the client. This token can be generated using a symmetric key algorithm or an asymmetric key algorithm. On each subsequent request after a successful login, the client will send a generated token back to the server. The server will check whether the sent token is valid or not and also checks whether its expired or not. The client will send this token in Authentication Bearer header.
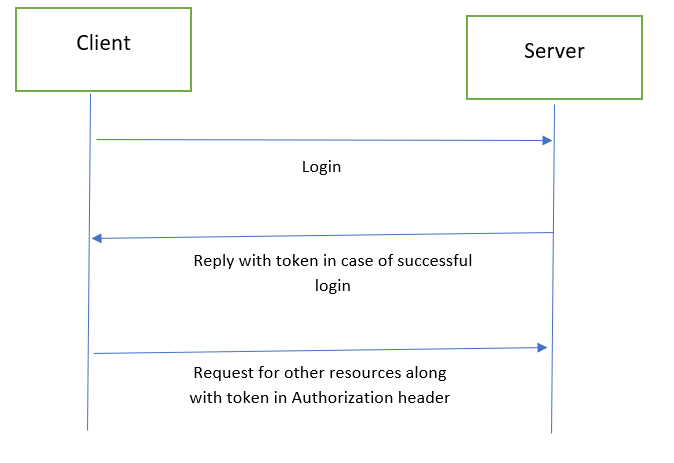
JWT token has a particular format. Header, Payload, and Signature.
- Header
- We need to specify which token system we want to use and also need to specify the algorithm type.
- Payload
- This is a token body. Basically, it contains expiry detail, claims details, issuer detail, etc.
- Signature
- To create the signature part we have to take the encoded header, the encoded payload, a secret, the algorithm specified in the header, and sign that.
E.g. HMACSHA256( base64UrlEncode(header) + "." + base64UrlEncode(payload), secret)
Benefits of using JWT
- JSON parser is common in programming languages.
- Secure. We can use a Symmetric or Asymmetric key algorithm.
- Less verbose in comparison to SAML.
I have created an ASP.Net Core web API sample application. JWTAuthService is responsible for the generation and validation of token. Feel free to download and contribute to the code on Github.
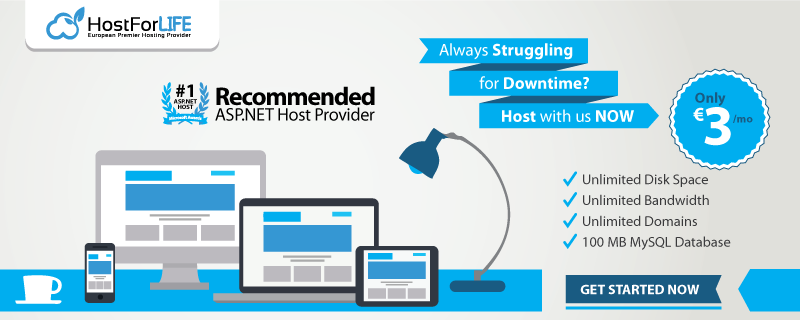