
December 22, 2020 09:10 by
Peter
This article provides some guidelines on how to use AutoMapper in C#.
We have come across a lot of situations when we need to copy data from one object to another. Normally, we follow the below mentioned approach to achieve this. Let’s say we have two classes declared as below,
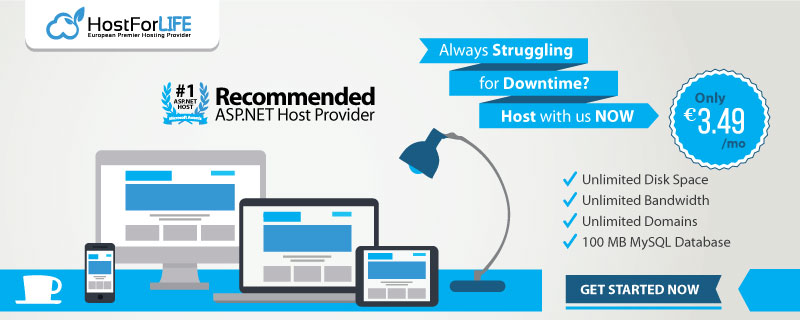
First Class:
public class UserObject
{
public int Id;
public string Name;
public string Address;
}
Second Class:
public class UserAnotherObject
{
public int Id;
public string Name;
public string Address;
}
Now, if an object of the first class have some data in it and we want to copy that data to an object of the second class, we would be using the following approach for this:
UserObject uObj = new UserObject(); //an object of First Class
UserAnotherObject uAnotherObj = new UserAnotherObject(); //an object of Second
uAnotherObj.Id = uObj.Id;
uAnotherObj.Name = uObj.Name;
uAnotherObj.Address = uObj.Address;
This is undoubtedly a tedious and repetitive task in case the object has a lot of properties.
Now, to overcome this task we can use a Nuget Package called the Auto-Mapper. Its working is quite simple to explain, in that it creates copy of one object into another but a bit automatically on the basis of Datatypes and names of the properties.
In order to use AutoMapper we would have to install it first from the Nuget Package Manager as pictorially explained below:
Step 1: Go to Manage NuGet Packages.. option in the project.
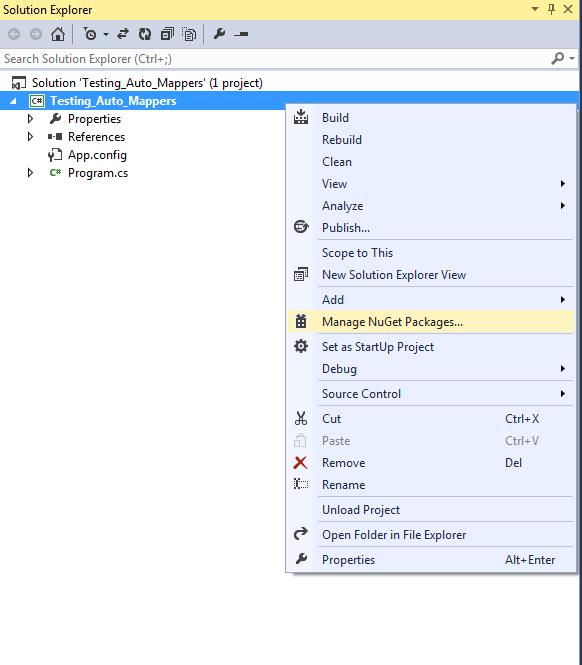
Step 2: Search and install AutoMapper to your project.
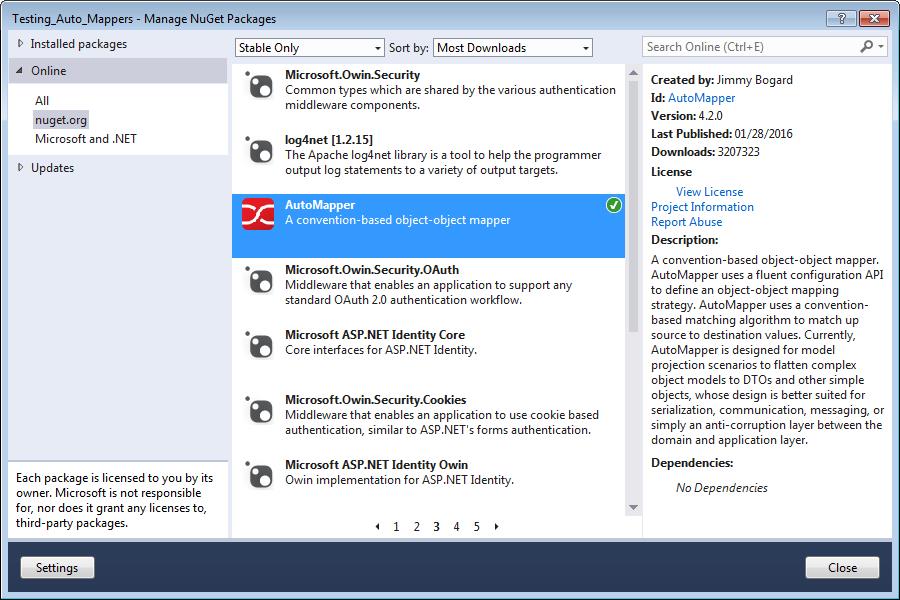
Step 3: Include the required Library on the page.
using AutoMapper.Mappers;
Now that this been done, we create a Mapper between the UserObject class and UserAnotherObject class. This will be done using the following line.
AutoMapper.Mapper.CreateMap<UserObject, UserAnotherObject>();
After creating this Mapper, let’s put some data into the object of the class UserObject that we made above,
uObj.Id = 123;
uObj.Name = "Peter";
uObj.Address = "London";
Now that we have the data, let’s copy the data from the object of UserObject class into the object of class UserAnotherObject using the AutoMapper that we just created above.
uAnotherObj = AutoMapper.Mapper.Map<UserAnotherObject>(uObj);
This copies the complete data from the uObj object to uAnotherObj object.
Note:
We need to keep it in mind that Auto Mapper copies data to properties in the target object with the same name as the properties name in the source object. The name should be the same but NOT CASE-SENSITIVE i.e. the name in source object can be “id” and that in the target object can be “ID”.