
January 9, 2024 06:24 by
Peter
Cross-Origin Resource Sharing (CORS) is a vital security feature that controls how resources on a web page can be accessed by web applications from different domains. In an ASP.NET Core project, enabling CORS involves configuring the server to allow or restrict access to its resources from different origins. Here's a step-by-step guide on establishing CORS in your ASP.NET Core application.
Step 1. Set up the middleware for CORS
Installing the Microsoft.AspNetCore.Cors package is the first step. Using the NuGet Package Manager Console, you may accomplish this.
Install-Package Microsoft.AspNetCore.Cors
Alternatively, you can add it to your project's .csproj file:
<PackageReference Include="Microsoft.AspNetCore.Cors" Version="x.x.x" />
Replace x.x.x with the latest version available.
Step 2. Configure CORS in Startup.cs
Open your Startup.cs file and locate the ConfigureServices method. Add the CORS service by calling AddCors in the ConfigureServices method.
public void ConfigureServices(IServiceCollection services)
{
// Other configurations
services.AddCors(options =>
{
options.AddPolicy("AllowSpecificOrigin",
builder =>
{
builder.WithOrigins("https://example.com")
.AllowAnyHeader()
.AllowAnyMethod();
});
});
// Other configurations
}
In the code snippet above:
- The CORS services are added to the application's service container via AddCors.
- AddPolicy generates a specified CORS policy that identifies permitted origins, headers, and methods ("AllowSpecificOrigin" in this example).
To designate which domains are permitted to access your resources, modify WithOrigins. To accept requests from any origin, use a "*".
Step 3. Turn on the CORS middleware
Add the CORS middleware to Startup.cs's Configure function.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Other configurations
app.UseCors("AllowSpecificOrigin");
// Other configurations
}
This middleware must be added before other middleware that may handle requests, such as MVC or static file middleware.
Step 4: Verify Your CORS Setup
Once set up, make queries to your ASP.NET Core APIs from various origins to test the CORS settings. Make that the methods, headers, and permitted origins meet the needs of your application.
In summary
For secure communication between your application and clients from different domains, and to control access to your resources, you must implement CORS in your ASP.NET Core project. You can increase the security of your application while enabling critical cross-origin communication by defining CORS policies, which allow you to control which origins can access your APIs.
Always keep in mind to properly set your CORS policies based on the security requirements of your application, keeping in mind the possible hazards related to cross-origin requests.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
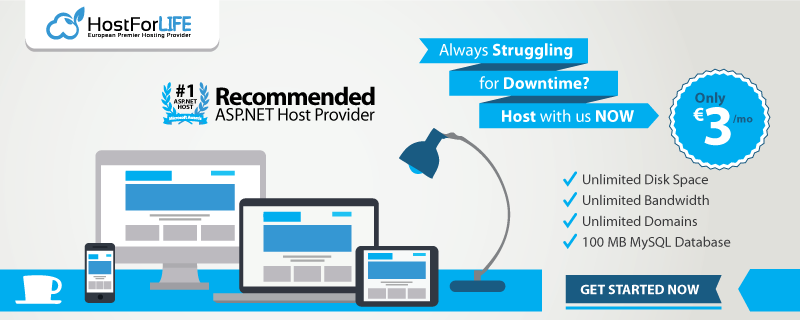