Microsoft's open-source web framework, ASP.NET Core, provides developers with a versatile and modular platform for developing high-performance web applications. One of the most important components of ASP.NET Core application development is effectively managing dependencies. AddTransient and AddScoped are two often used techniques for registering services in the world of dependency injection, particularly when working with repositories. Choosing between these strategies can have a major impact on your application's behavior and performance. This detailed guide seeks to clarify the distinctions between AddTransient and AddScoped in order to assist developers in making educated decisions when registering repositories in ASP.NET Core apps.
Understanding ASP.NET Core Dependency Injection
DI is a design pattern that encourages loose coupling between components of an application by allowing one object to satisfy the dependencies of another. DI is a critical method in ASP.NET Core for controlling object instantiation and lifespan.
AddTransient: Short-lived Instances
AddTransient is used to register services that are created each time they are requested. In the context of repository registration, this means a new instance of the repository is created every time it is injected into a component such as a controller or a service. Transient services are suitable for lightweight, stateless services or repositories that don’t store any client-specific data.
services.AddTransient<IRepository, Repository>();
AddScoped: Scoped Instances
In contrast, AddScoped generates a single instance of the service for each scope. Each HTTP request generates a scope in the context of web applications. This means that all components sharing the same scoped instance of a service will use the same instance of that service within a single HTTP request. Scoped services are useful for stateful components such as database contexts or repositories that require state to be maintained across several areas of the application within a single request.
services.AddScoped<IRepository, Repository>();
Selecting AddTransient vs. AddScoped
The decision between AddTransient and AddScoped comes down to the desired behavior of your repositories:
When should you use AddTransient?
Statelessness is essential: Using AddTransient is useful if your repository is stateless and does not save any data between method calls. Each method call is assigned a repository instance.
Transient services are appropriate for lightweight operations when the expense of producing a new instance is small in comparison to the benefits of having a fresh instance each time.
Use AddScoped When:
Stateful Operations: If your repository or service maintains state throughout the duration of an HTTP request, using AddScoped ensures that all parts of your application that need the repository within the same request share the same instance.
Database Contexts: When dealing with Entity Framework Core’s DbContext, using AddScoped is crucial. DbContext instances should typically be scoped to the lifetime of a request to ensure data consistency and proper unit of work pattern.
Performance and Memory Usage Considerations
AddTransient is often faster in terms of performance because it does not use tracking scopes. However, in most situations, the difference may be minimal, and the ease and safety afforded by AddScoped frequently outweigh any minor performance improvements.
In terms of memory utilization, AddTransient can cause a greater number of instances to be created, thereby straining memory resources, particularly in high-traffic applications. AddScoped can improve memory usage by reusing instances inside the scope of a request.
The choice between AddTransient and AddScoped for repository registration in ASP.NET Core is determined by your application's individual requirements and characteristics. Understanding the fundamental distinctions and consequences of these technologies is critical for developing efficient, high-performance, and maintainable online applications. You can ensure that your services and repositories operate as intended by selecting the proper lifespan method, resulting in a seamless user experience and optimal resource use.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
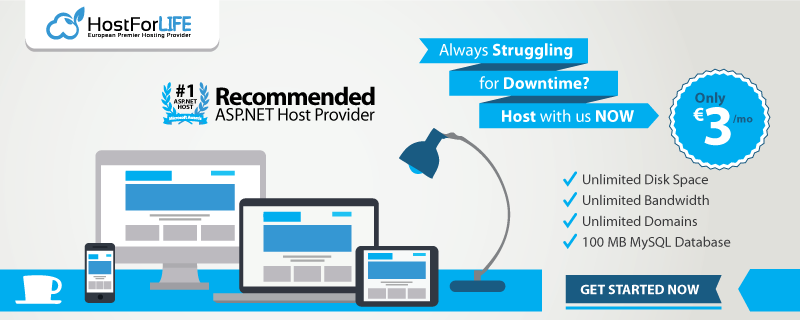