
November 22, 2021 09:05 by
Peter
Log4net is an amazing library to perform logging in our applications in a petty simple way.
To start with log4net in ASP.NET we need to install the nuget package.
dotnet add package log4net
Then we need to include the log4net.config file. This is an XML file that contains all the settings for our Log. We can specify the format, filename, maximum file size, and others.
This is an example,
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<log4net>
<root>
<level value="ALL" />
<appender-ref ref="RollingLogFileAppender" />
</root>
<appender name="RollingLogFileAppender" type="log4net.Appender.RollingFileAppender">
<file value="api.log" />
<appendToFile value="true" />
<rollingStyle value="Composite" />
<maxSizeRollBackups value="5" />
<maximumFileSize value="10MB" />
<layout type="log4net.Layout.PatternLayout">
<conversionPattern value="%date %5level %class - MESSAGE: %message%newline"/>
</layout>
</appender>
</log4net>
</configuration>
Markup
file: Name of the file created with the log
level: Used to Specify the level error to save in the log. Other levels are going to be ignored and ALL includes all the levels.
maximumFileSize: Maximum size of the log file if the log exceeds this limit, log4net is going to create a new file.
This is an example where the file is located,
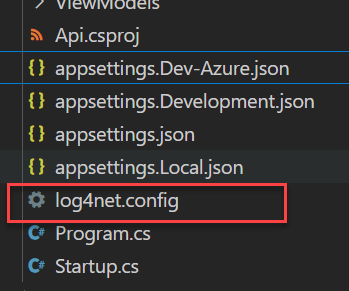
Now, we need to set up the log in the program.cs class to start the log when the API starts.
public static void Main(string[] args)
{
var host = CreateHostBuilder(args).Build();
ILoggerRepository repository = log4net.LogManager.GetRepository(Assembly.GetEntryAssembly());
var fileInfo = new FileInfo(@"log4net.config");
log4net.Config.XmlConfigurator.Configure(repository, fileInfo);
host.Run();
}
Finally, we are ready to create a filter in our project in order to catch all the possible exceptions in our application.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using log4net;
using Microsoft.AspNetCore.Mvc.Filters;
using Microsoft.Extensions.Logging;
namespace Api.Attributes
{
public class LogFilterAttribute : ExceptionFilterAttribute
{
ILog logger;
public LogFilterAttribute()
{
logger = LogManager.GetLogger(typeof(LogFilterAttribute));
}
public override void OnException(ExceptionContext Context)
{
logger.Error(Context.Exception.Message + " - " + Context.Exception.StackTrace);
}
}
}
Add to the services the filter created:
services.AddControllersWithViews(p=> p.Filters.Add(new LogFilterAttribute()));
Now, all the exceptions are going to be saved in the log file. This log is on Api\bin\Debug\net5.0

Log Example

You still can use the .NET Logger class when you want to log something manually.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
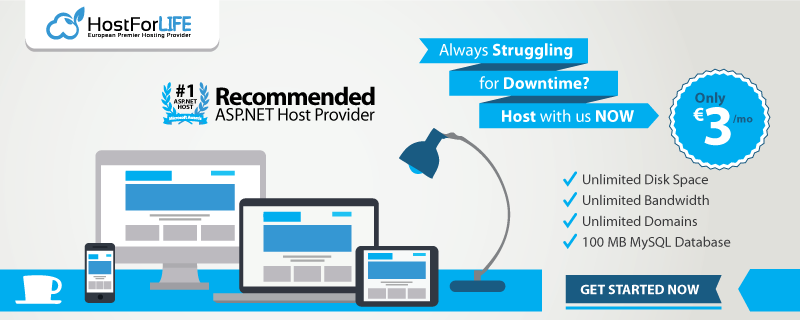