
March 20, 2023 08:46 by
Peter
In today's fast-paced world of software development, it's essential to have reliable and resilient applications. With the rise of cloud computing and microservices, network failures and system errors are inevitable. In such scenarios, retrying the failed operations is a common solution to keep the application up and running. Polly is a powerful .NET library that provides a flexible and easy-to-use solution to handle such scenarios.
This article will discuss how to use Polly in .NET 6 to handle network failures, retries, and circuit breakers.
What is Polly?
Polly is an open-source library for .NET that provides a simple and elegant way to handle transient errors, retries, and circuit breakers. It allows developers to define policies to handle different types of exceptions and failures. Polly is flexible, extensible, and easy to use, making it an excellent choice for building resilient applications.
Getting started with Polly
To use Polly in your .NET 6 application, you must add the Polly NuGet package to your project. You can do this by using the NuGet Package Manager or adding the package reference to the .csproj file.
Once you have added the Polly package to your project, you can use it in your code. Here's a simple example of how to use Polly to handle a network failure and retry the operation.
using Polly;
using System;
using System.Net.Http;
public static async Task < string > GetResponseData(string url) {
var policy = Policy.Handle < HttpRequestException > ().WaitAndRetryAsync(3, retryAttempt => TimeSpan.FromSeconds(Math.Pow(2, retryAttempt)));
var client = new HttpClient();
var response = await policy.ExecuteAsync(async () => await client.GetAsync(url));
return await response.Content.ReadAsStringAsync();
}
In the above code, we define a policy that handles HttpRequestException and retries the operation up to three times with an exponential backoff strategy. The WaitAndRetryAsync method takes two parameters: the number of retries and a function that calculates the delay between retries.
We then create an instance of the HttpClient and use the ExecuteAsync method of the policy to execute the GET request. Polly will automatically retry the operation according to the defined policy if the request fails due to a network failure.
Handling Circuit Breakers
Circuit breakers are another important aspect of building resilient applications. They prevent an application from repeatedly making requests to a failing service, which can overload the system and cause it to crash.
Polly provides an easy-to-use circuit breaker implementation that allows you to define a threshold for failed operations. Once the threshold is reached, the circuit breaker will trip and stop executing requests for a specified amount of time.
Here's an example of how to use Polly's circuit breaker,
var policy = Policy
.Handle<HttpRequestException>()
.CircuitBreakerAsync(
handledEventsAllowedBeforeBreaking: 2,
durationOfBreak: TimeSpan.FromSeconds(30)
);
try
{
await policy.ExecuteAsync(async () => await client.GetAsync(url));
}
catch (Exception ex)
{
// Handle the exception
}
In the above code, we define a policy that handles HttpRequestException and trips the circuit breaker after two consecutive failures. The circuit breaker remains open for 30 seconds, after which it resets and allows requests to be executed again.
In conclusion, Polly is a powerful library that provides a flexible and easy-to-use solution for handling network failures, retries, and circuit breakers. Polly's simple and elegant API makes it easy to build resilient applications that can handle transient errors and keep running under adverse conditions. By using Polly in your .NET 6 application, you can make your application more reliable, and scalable.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
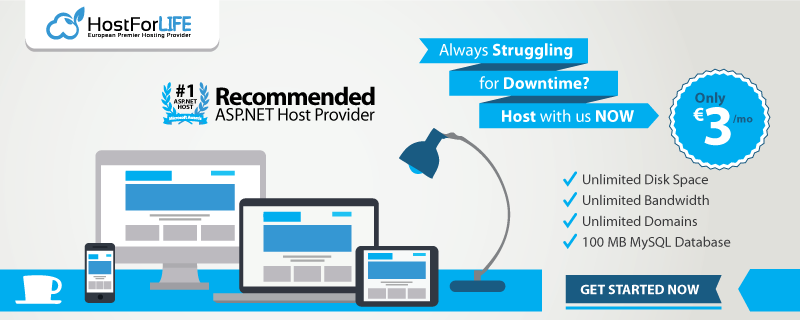