
December 5, 2024 07:36 by
Peter
In Visual Studio 2022 or 2019, create a .Net Worker service project and give it whatever name you like. The worker service was first implemented in Visual Studio 2019 and is compatible with the most recent versions. A worker service is a background service that performs ongoing background tasks while operating as a service.
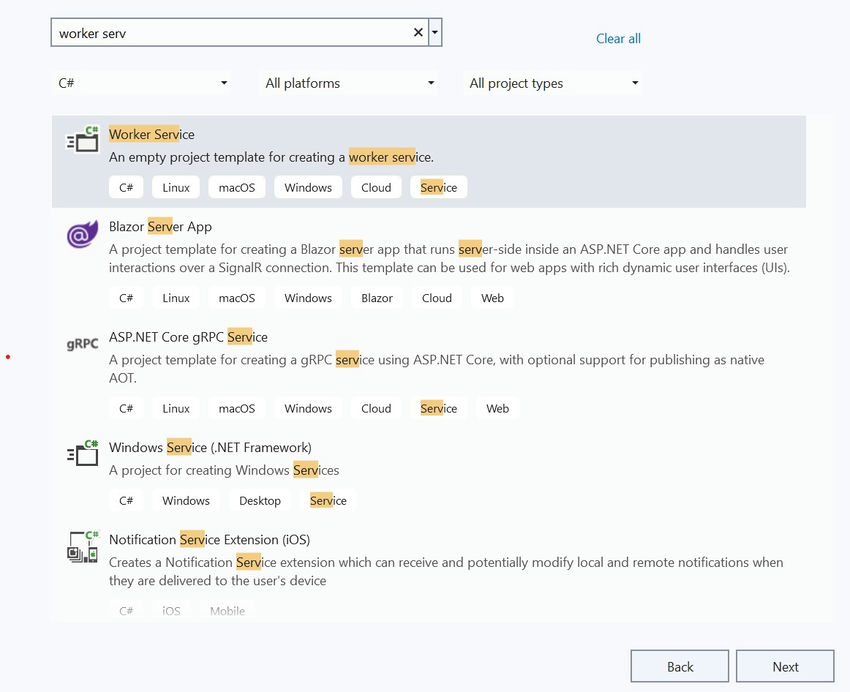
Worker.cs
namespace MyWorkerService
{
public class Worker : BackgroundService
{
private readonly ILogger<Worker> _logger;
public Worker(ILogger<Worker> logger)
{
_logger = logger;
}
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
while (!stoppingToken.IsCancellationRequested)
{
if (_logger.IsEnabled(LogLevel.Information))
{
_logger.LogInformation("Worker running at: {time}", DateTimeOffset.Now);
}
await Task.Delay(1000, stoppingToken);
}
}
}
}
To install the Worker service in Windows, the background service installs the below-mentioned Hosting package from the Nuget package.
Microsoft.Extensions.Hosting.WindowsServices
Add the code below to the program.cs
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.UseWindowsService()
.ConfigureServices((hostContext, services) =>
{
services.AddHostedService<YourWorkerService>();
});
Build and Publish the worker service project and make sure MyWorkerService.exe is placed inside the publish folder. Then, Open the Command prompt with administrative privileges and execute the mentioned command lines.
sc.exe create MyWorkerService binpath=C:\MyWorkerService\MyWorkerService.exe
sc.exe start MyWorkerService
In case of any error, if you want to delete or stop a service, follow the command lines.
sc.exe delete MyWorkerService
sc.exe stop MyWorkerService
To ensure the smooth running of the worker service, open a service from the start of a window, check the name of the service (Example: MyWorkerService), and confirm the service is running.
Output
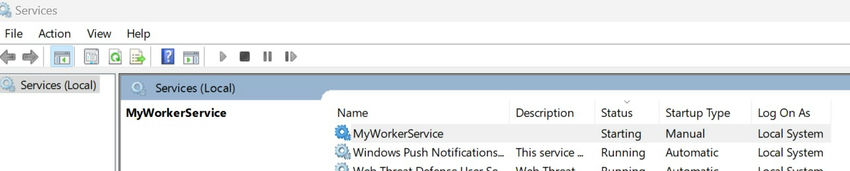
European Best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
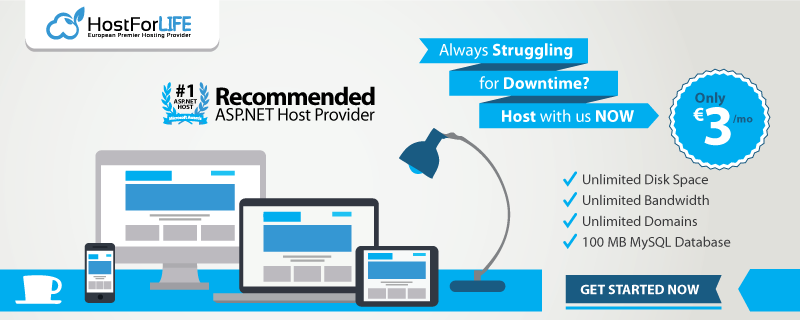