
January 19, 2021 08:26 by
Peter
A special method of the class that is automatically invoked when an instance of the class is created is called a constructor. A constructor is a special method that is used to initialize objects. The advantage of a constructor, is that it is called when an object of a class is created.
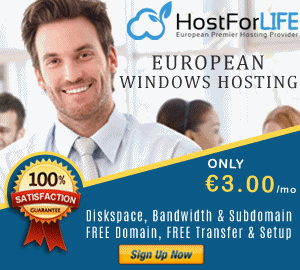
When we create instance of a class a default constructor is invoked automatically having the same name as Class . Some key points,
Constructor is used to initialize an object (instance) of a class.
Constructor is a like a method without any return type.
Constructor has the same name as class name.
Constructor follows the access scope (Can be private, protected, public, Internal and external).
Constructor can be overloaded.
Following is the list of constructors in C#.
Default constructor
Parameterized constructor
Private Constructor
Static Constructor
Copy Constructor
Default Constructor
A constructor without any parameters is called a default constructor. If we do not create constructor the class will automatically call default constructor when an object is created.
Example of Default Constructor,
using System;
namespace DefaultConstractor {
class addition {
int a, b;
public addition() //default contructor
{
a = 100;
b = 175;
}
public static void Main() {
addition obj = new addition(); //an object is created , constructor is called
Console.WriteLine(obj.a);
Console.WriteLine(obj.b);
Console.Read();
}
}
}
Parameter Constructor
A constructor with at least one parameter is called a parameterized constructor.
Private Constructor
Private constructors are used to restrict the instantiation of object using 'new' operator. A private constructor is a special instance constructor. It is commonly used in classes that contain static members only.
Static Constructor
A static constructor is used to initialize any static data, or to perform a particular action that needs to be performed once only. It is called automatically before the first instance is created or any static members are referenced
Copy Constructor
The constructor which creates an object by copying variables from another object is called a copy constructor.
Is it possible to have a class without Constructor?
If a class contains no instance constructor declarations, a default instance constructor is automatically provided. The only classes in C# which don't have any instance constructors are static classes, and they can't have constructors.
Example
class Person {
//This class has no constructor
public void getPersonProfile() {
Console.WriteLine("Base class:getPersonProfile() have no constructor");
}
}
class Program {
static void Main(string[] args) {
//Create object of person
Person p = new Person();
p.getPersonProfile();
}
}
HostForLIFE ASP.NET 3.1.9 Hosting
HostForLIFE is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.