In this tutorial, we will learn how to call a Power Automate flow from .NET, using an HTTP post. For this purpose, we will use an example to send an email.
This is the flow created in Power Automate:
Power Automate Flow
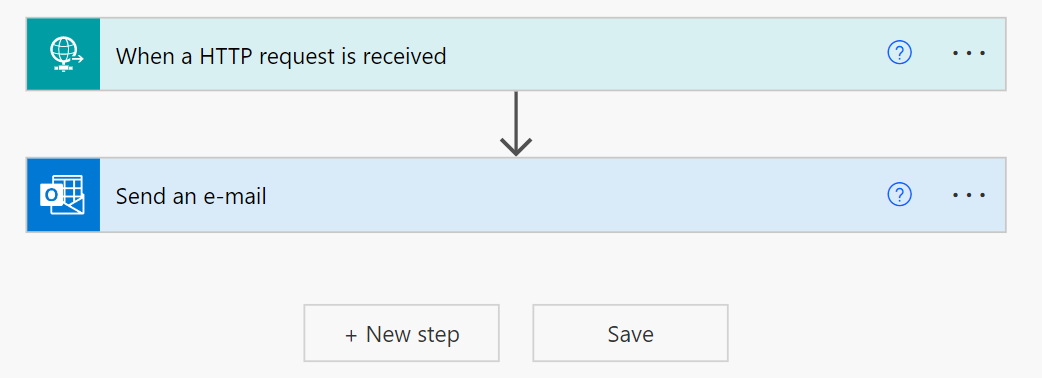
1. .NET Development
In the first instance, we must refer to the endpoint of our Power Automate flow:
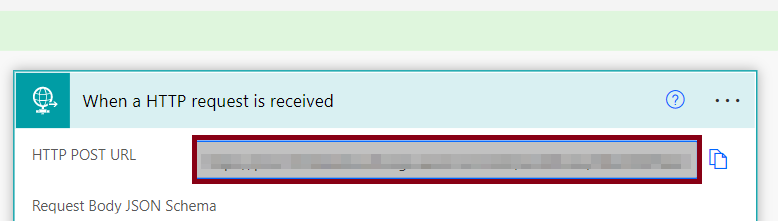
private string uri = "HTTP POST URL";
Then we can define a method in a service for example to be able to call it from somewhere in our application, and this will receive as parameters the username, toAddress, and the emailSubject. The idea with this method is to be able to prepare the request for the HTTP post, and that the Power Automate flow can be triggered:
public async Task SendEmailAsync(string username, string toAddress, string emailSubject)
{
try
{
HttpClient client = new HttpClient();
client.BaseAddress = new Uri(uri);
client.DefaultRequestHeaders.Accept.Clear();
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Post, client.BaseAddress);
var body = $"{{\"emailAddress\": \"{toAddress}\",\"emailSubject\":\"{emailSubject}\",\"userName\":\"{username}\"}}";
var content = new StringContent(body, Encoding.UTF8, "application/json");
request.Content = content;
var response = await MakeRequestAsync(request, client);
Console.WriteLine(response);
}
catch (Exception e)
{
Console.WriteLine(e.Message);
throw new Exception();
}
}
Finally, we can count on an additional method to be able to perform the HTTP post request, according to the previously established request:
public async Task<string> MakeRequestAsync(HttpRequestMessage getRequest, HttpClient client)
{
var response = await client.SendAsync(getRequest).ConfigureAwait(false);
var responseString = string.Empty;
try
{
response.EnsureSuccessStatusCode();
responseString = await response.Content.ReadAsStringAsync().ConfigureAwait(false);
}
catch (HttpRequestException)
{
// empty responseString
}
return responseString;
}
2. Test the code in a web app with ASP.NET
To perform the tests, let's see an example in a web application from ASP.NET that calls this deployed Power Automate service, when a user is added from a form, and notified by email with this flow:
await new PowerAutomateService().SendEmailAsync(student.FirstName, student.Email, "Greetings from ASP.NET / DotVVM web application.");
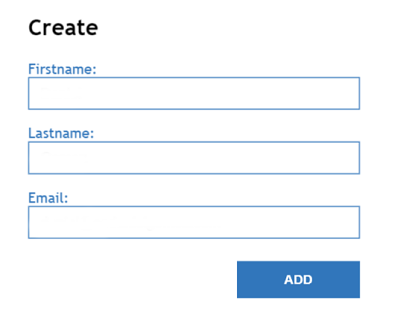
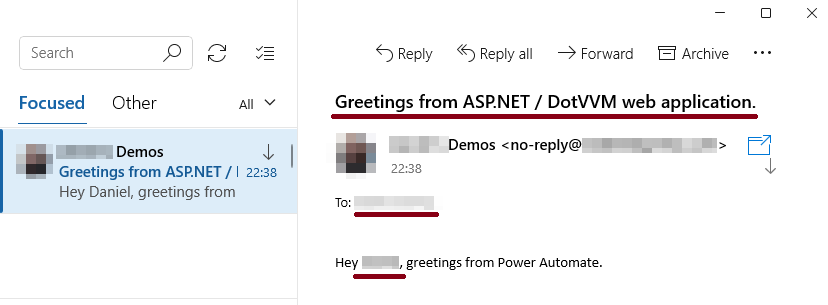
Thanks for reading.
I hope you liked the article. If you have any questions or ideas in mind, it'll be a pleasure to be able to communicate with you and together exchange knowledge with each other.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
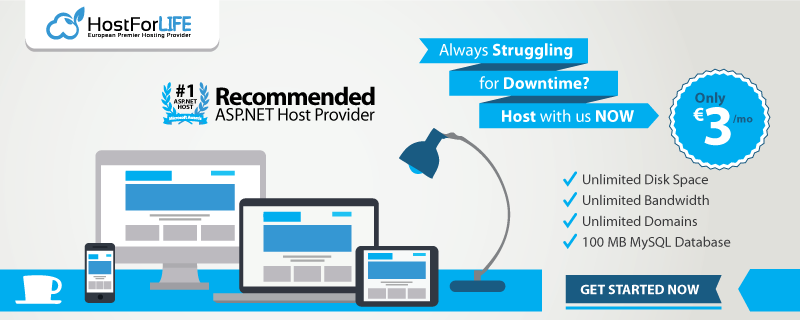