
March 26, 2024 08:05 by
Peter
Many current software applications rely on background processes or services to complete numerous functions asynchronously without interfering with the user experience. Background services, whether they're processing data, sending emails, or conducting routine maintenance activities, are critical to keeping applications responsive and efficient. Background services in the.NET Core ecosystem make it easy and efficient to implement asynchronous operations.
What are Background Services?
Background services in.NET Core are long-running processes that operate independently of the main application thread. They run in the background and often conduct activities like data processing, monitoring, or periodic actions without interfering with the main application's execution flow. These services are built on the BackgroundService base class supplied by the.NET Core framework, making it easy to manage their lifecycle and execution.
Benefits of Background Services
- Improved Performance: By offloading tasks to background services, the main application thread remains responsive, providing a smoother user experience.
- Scalability: Background services can be scaled independently, allowing applications to handle varying workloads efficiently.
- Asynchronous Processing: Background services enable asynchronous processing of tasks, enabling applications to perform multiple operations concurrently.
- Modular Design: Separating background tasks into services promotes modular and maintainable code, enhancing the overall codebase's readability and manageability.
Implementing Background Services in.NET Core
Let's look at an example to learn how to develop background services in.NET Core.
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Logging;
using System;
using System.Threading;
using System.Threading.Tasks;
public class ExampleBackgroundService : BackgroundService
{
private readonly ILogger<ExampleBackgroundService> _logger;
public ExampleBackgroundService(ILogger<ExampleBackgroundService> logger)
{
_logger = logger;
}
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
while (!stoppingToken.IsCancellationRequested)
{
_logger.LogInformation("Background service is running at: {time}", DateTimeOffset.Now);
// Perform your background task here
await Task.Delay(5000, stoppingToken); // Delay for 5 seconds before the next iteration
}
}
}
In this example
- We create a class ExampleBackgroundService that inherits from BackgroundService.
- In the constructor, we inject an instance of ILogger to log messages.
- We override the ExecuteAsync method, where the actual background task logic resides. Inside this method, we have a loop that runs until cancellation is requested.
- Within the loop, we perform the background task, in this case, logging the current time.
- We use Task. Delay to introduce a 5-second delay before the next iteration.
Registering Background Services
To use background services in your .NET Core application, you need to register them with the Dependency Injection container in your Startup. cs file.
public void ConfigureServices(IServiceCollection services)
{
services.AddHostedService<ExampleBackgroundService>();
}
Background services in .NET Core offer a powerful mechanism for implementing asynchronous tasks in applications. By leveraging the BackgroundService base class, developers can easily create and manage long-running background tasks, enhancing application responsiveness and scalability. With the provided example and insights, you should now have a solid understanding of how to implement and utilize background services effectively in your .NET Core applications.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
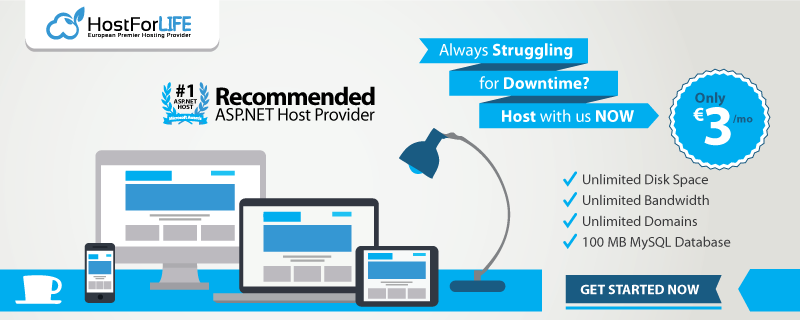