
November 21, 2024 06:35 by
Peter
CountBy, a new LINQ function introduced in.NET 9, simplifies common data grouping and counting operations. By effectively classifying elements according to a given key, this function generates a collection of KeyValuePair objects. Every key-value pair denotes a group and the number of elements that go with it. Before.NET 9, we had to use a two-step procedure to group elements and count how often they appeared. First, we categorized elements according to a particular key using the GroupBy technique. We then projected each group in order to determine how many elements it contained.
Example
var message = "welcometoprpcoding";
var characterOccurences = message
.GroupBy(c => c)
.Select(g => new { Key = g.Key, Value = g.Count() });
foreach (var characterOccurence in characterOccurences)
{
Console.WriteLine($"Character: {characterOccurence.Key}, " +
$"Count: {characterOccurence.Value}");
}
Grouping and Counting Characters
This code uses LINQ to group the characters in the string and count their occurrences.
- message.GroupBy(c => c): Groups the characters based on their value. Each group contains characters with the same value.
- Select(g => new { Key = g.Key, Value = g.Count() }): Projects each group into an anonymous object with two properties:
- Key: The character itself.
- Value: The count of occurrences of that character in the group.
Output
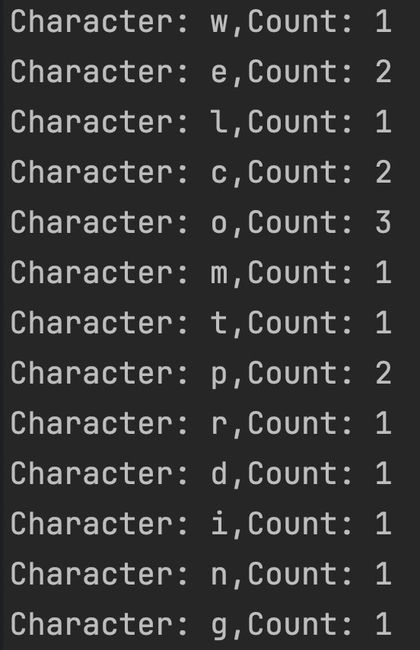
With .NET 9, the CountBy method significantly streamlines this process.
var message = "welcometoprpcoding";
var characterOccurences = message.CountBy(c => c);
foreach (var characterOccurence in characterOccurences)
{
Console.WriteLine($"Character: {characterOccurence.Key}, " +
$"Count: {characterOccurence.Value}");
}
Output
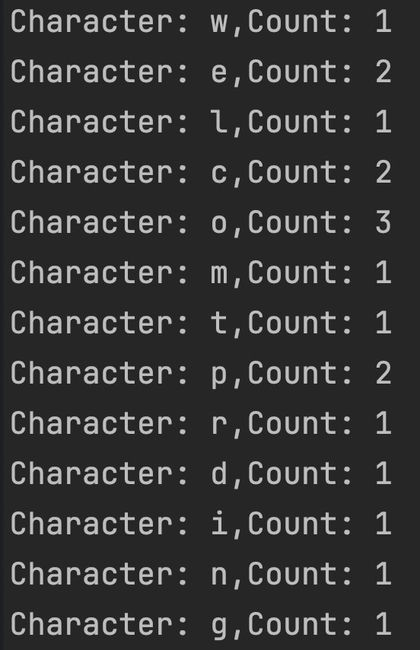
Key Benefits of CountBy
- Conciseness: Simplifies code, improving readability.
- Efficiency: Potentially optimizes performance by streamlining the grouping and counting process.
- Clarity: Clearly expresses the intent of categorizing and quantifying elements.
By leveraging the CountBy method, you can write more concise, efficient, and expressive LINQ queries in your .NET 9 applications. Happy Coding!
European Best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
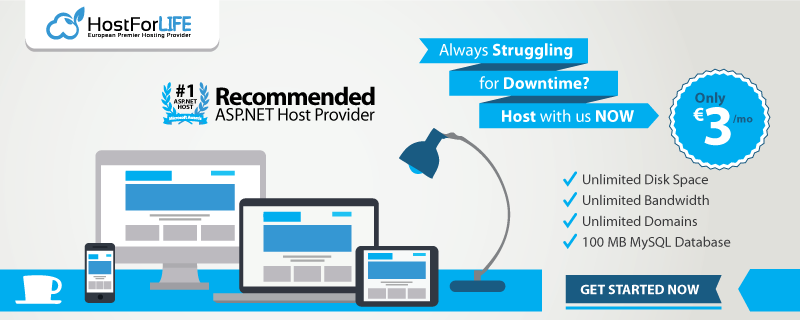