As a one-year developer, you've probably heard of the SOLID principles, which are a collection of five principles that promote clean, maintainable, and scalable code. The Single Responsibility Principle (SRP) is the most important of these concepts to understand and master. In this post, we'll describe SRP in layman's terms and present a simple.NET Core example to demonstrate its importance.
What exactly is SRP?
The Single Responsibility Principle (SRP) is the first letter in SOLID, and it emphasizes the need of keeping your code simple and well-organized. In a nutshell, SRP asserts that a class should have only one reason to modify. In other words, a class should only have one task or goal.
Consider real-world examples to help you understand this subject. Consider a simple kitchen equipment such as a toaster. Its primary function is to toast bread; it does not brew coffee or conduct any other unrelated duties. Similarly, classes in software development should have a single purpose.
What is the significance of SRP?
Readability: Classes having a single purpose are simpler to grasp for you and other developers, boosting code readability and maintainability.
Reusability: When a class excels at one task, it is more likely to be reusable in other parts of your application without producing unexpected side effects.
Smaller, more concentrated classes are easier to test. Specific tests for a class's single task can be written, making it easier to find and fix errors.
A Simplified.NET Core Example
Let's look at a simple.NET Core example to explain SRP. User Class. In this example, a User class is in charge of managing user-specific data such as name, email address, and age. This class's sole responsibility is to handle user data.
public class User
{
public string Name { get; set; }
public string Email { get; set; }
public int Age { get; set; }
public User(string name, string email, int age)
{
Name = name;
Email = email;
Age = age;
}
}
Classification of NotificationServices
Then we make a NotificationService class. This class is in charge of sending user notifications. It may send emails, SMS messages, or other types of communication. The key point is that it only has one responsibility: handling notifications.
public class NotificationService
{
public void SendEmail(User user, string message)
{
// Code to send an email notification
Console.WriteLine($"Email sent to {user.Name}: {message}");
}
public void SendSMS(User user, string message)
{
// Code to send an SMS notification
Console.WriteLine($"SMS sent to {user.Name}: {message}");
}
}
In this example, the User class deals with user data, while the NotificationService class manages sending notifications. This clear separation of responsibilities aligns with the Single Responsibility Principle.
Using the Classes
Here's how you can use these classes in your application.
public class Program
{
public static void Main()
{
var user = new User("Peter", "Peter@hfl.eu", 30);
var notificationService = new NotificationService();
// Sending a notification to the user
notificationService.SendEmail(user, "Hello, Peter! Don't forget about our meeting.");
notificationService.SendSMS(user, "Reminder: Meeting today at 3 PM.");
}
}
This example shows how to use the SRP to keep your code orderly, maintainable, and extensible. These concepts will assist a novice developer (fresher) in building more robust and scalable programs. With SRP, you'll be well on your way to being a more capable and efficient developer. Remember that in your journey as a software developer, simplicity and adherence to core principles such as SRP are critical to generating high-quality software.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
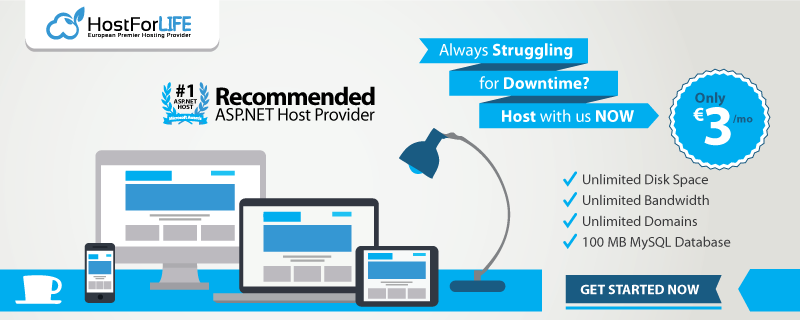