As we know there are so many changes in .Net 6 and C# 10. In this article, we will explore one of the changes in C#10 on the writing properties and objects of a class. More details on new features or changes in .NET 6 can be found in the previous article Features of .NET 6.
In .NET 5 and lower versions, we can write properties and objects of class like below.
public class Person {
public string Name;
public string Sex;
public string Address;
public string Email;
}
However, it has been changed in C# 10. If we write like earlier, the compiler shows a warning like below.
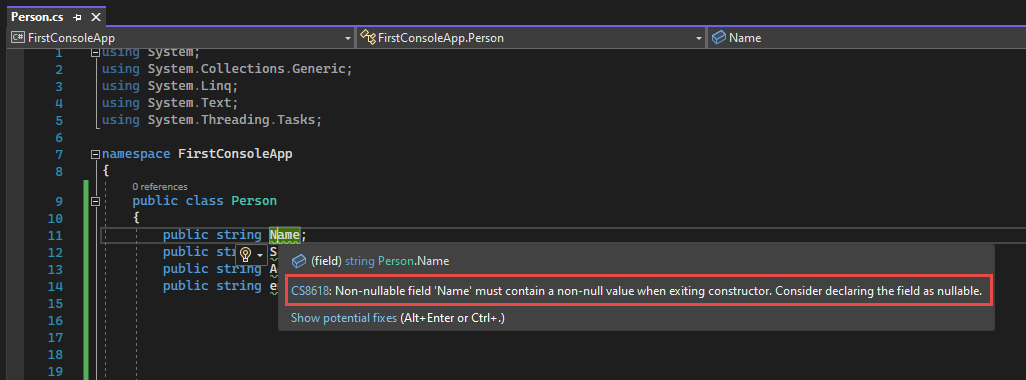
So, to handle this there are different ways. In this article, we will learn those methods to handle the warnings.
Let’s create a console application in .NET6
Create Console Application in .NET 6
Step 1
Open Visual Studio 2022 and click Create a new project.
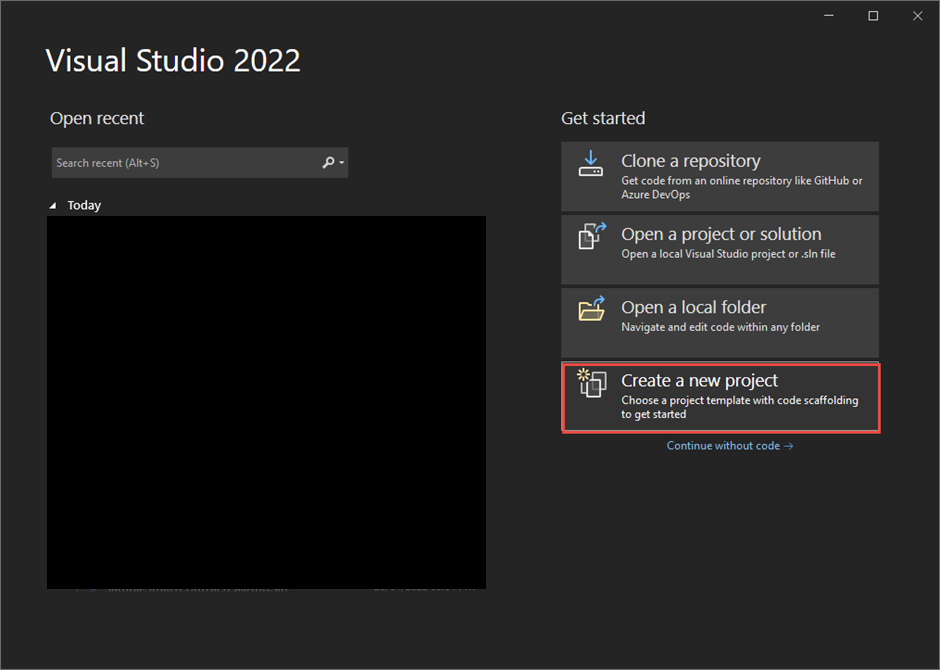
Step 2
Select Console App and click Next.
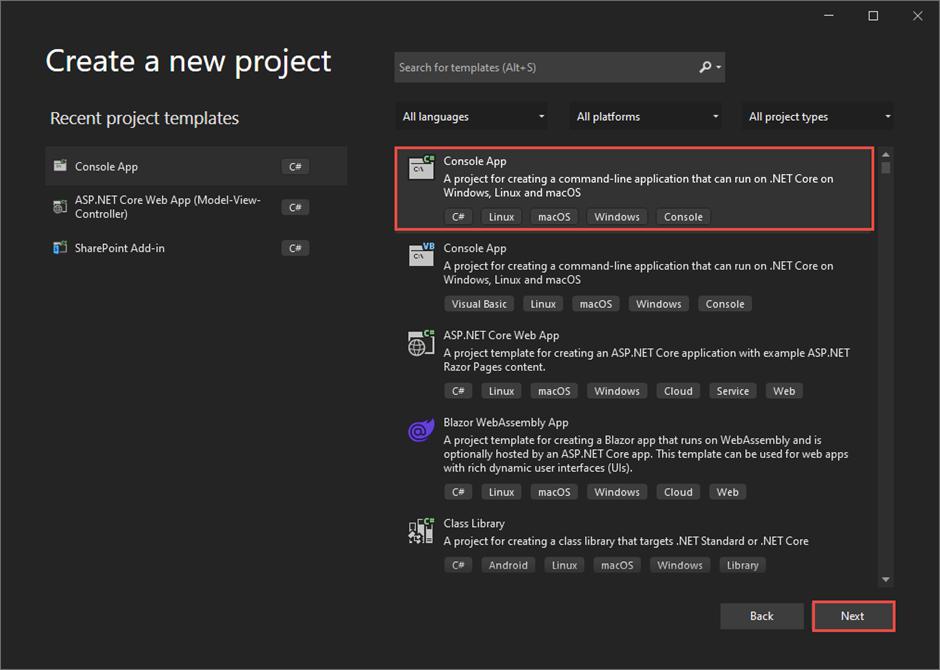
Step 3
Give the project name and location of the project.
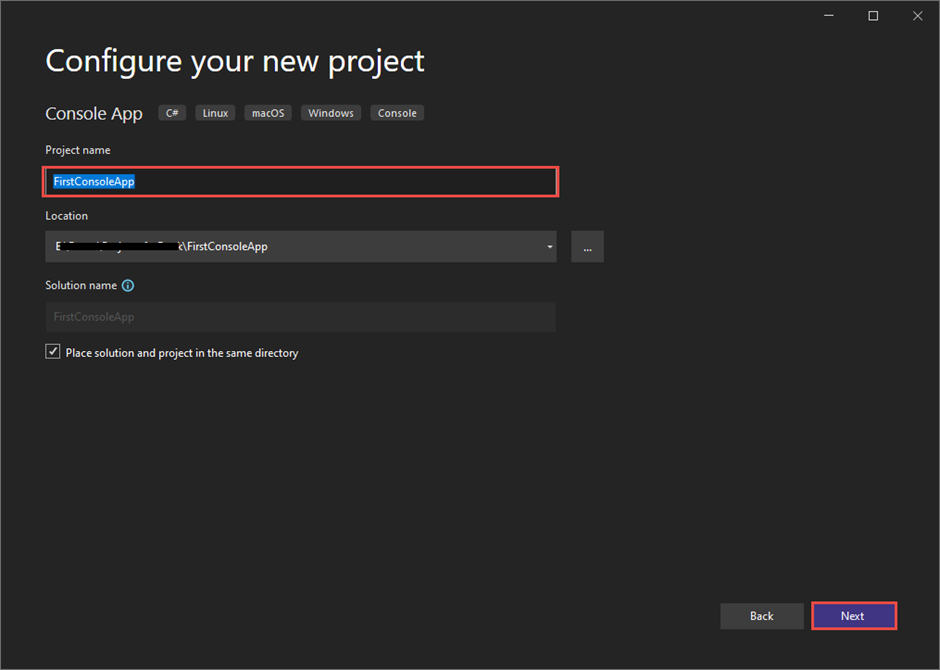
Step 4
Select framework: .NET 6.0 (Long-term support).
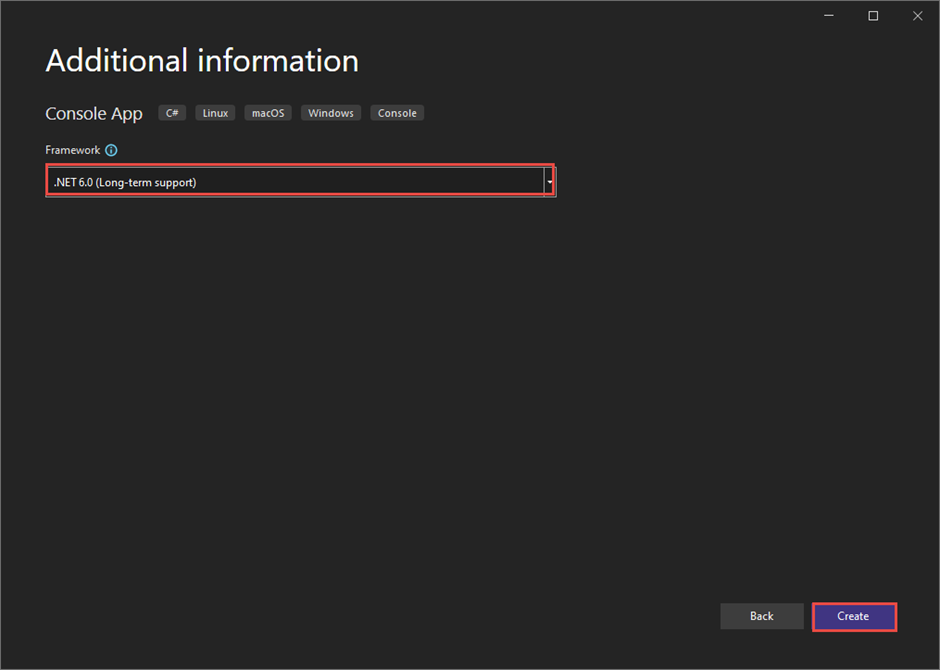
This creates the console app which looks like below.
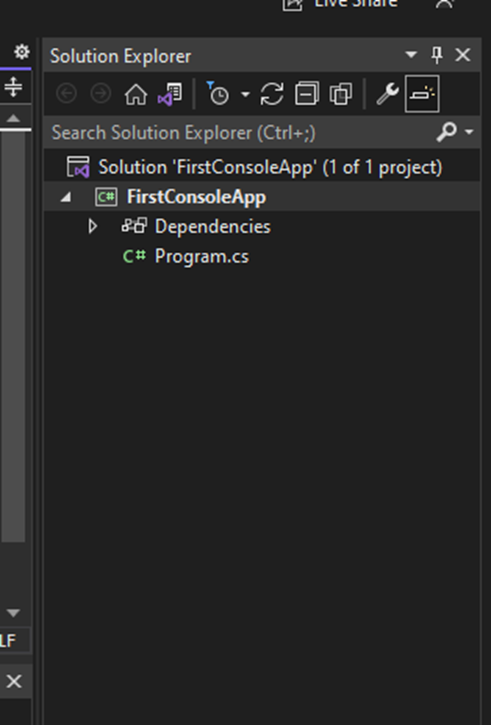
Default Program.cs file is listed below.
// See https://aka.ms/new-console-template for more information
Console.WriteLine("Hello, World!");
Now, let’s compile and run the program. when you run it, it will display the “Hello, World!” message. Now, we will move to the main point of this write-up.
Add a New Class
Right-click on project -> add class and give the name of the class as Person.
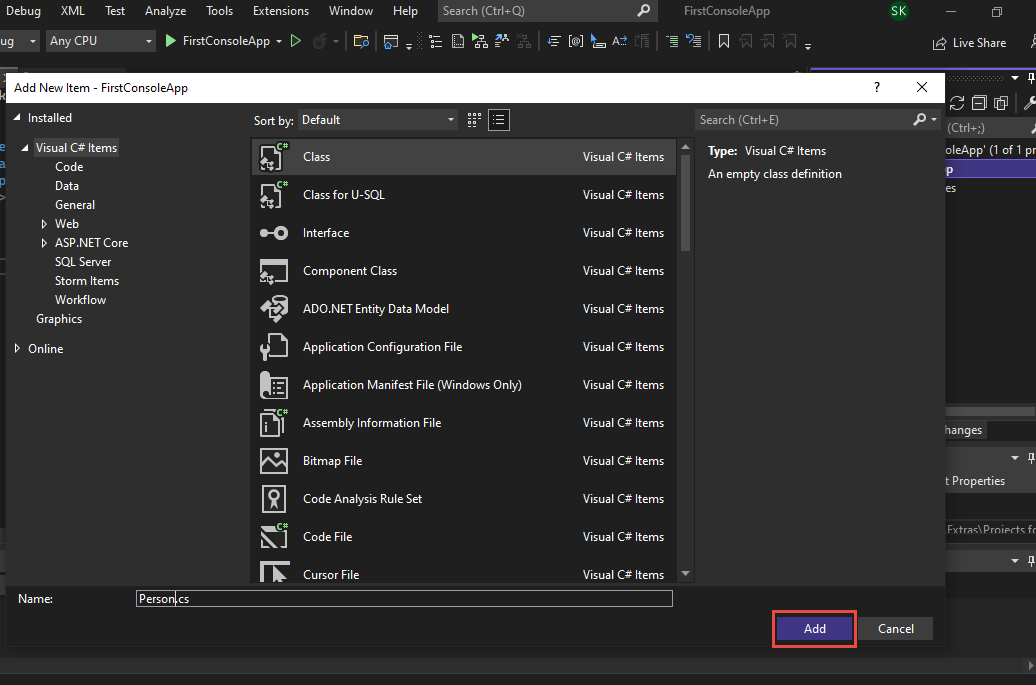
Person class contains the following properties. Write the below code.
public class Person {
public string Name;
public string Sex;
public string Address;
public string Email;
public string Description {
get;
set;
}
}
Then, you will get the warning as depicted below.
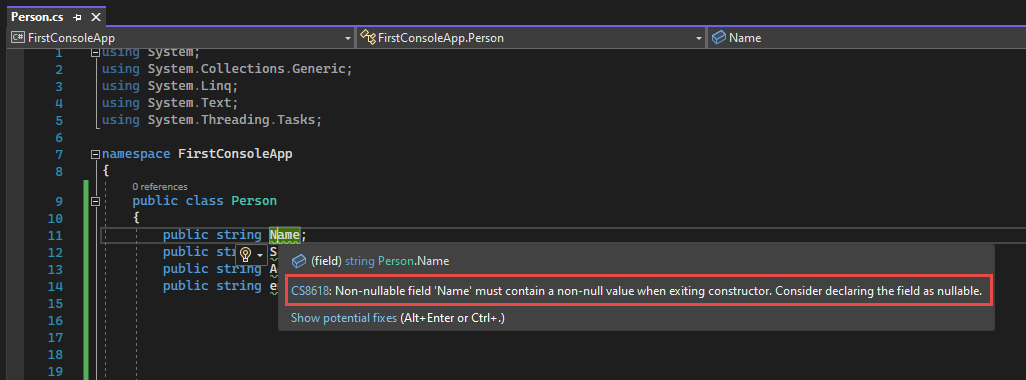
Let’s jump to different ways to handle the compiler warning.
Method 1
Changing the project file(project.csproj). You disable or remove the nullable in csproj file so that it will not show the warning message. Right-click on the project and Edit the project as shown below.
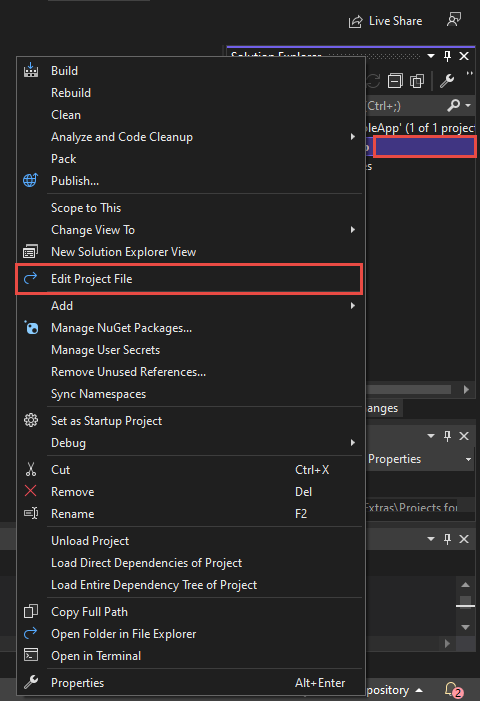
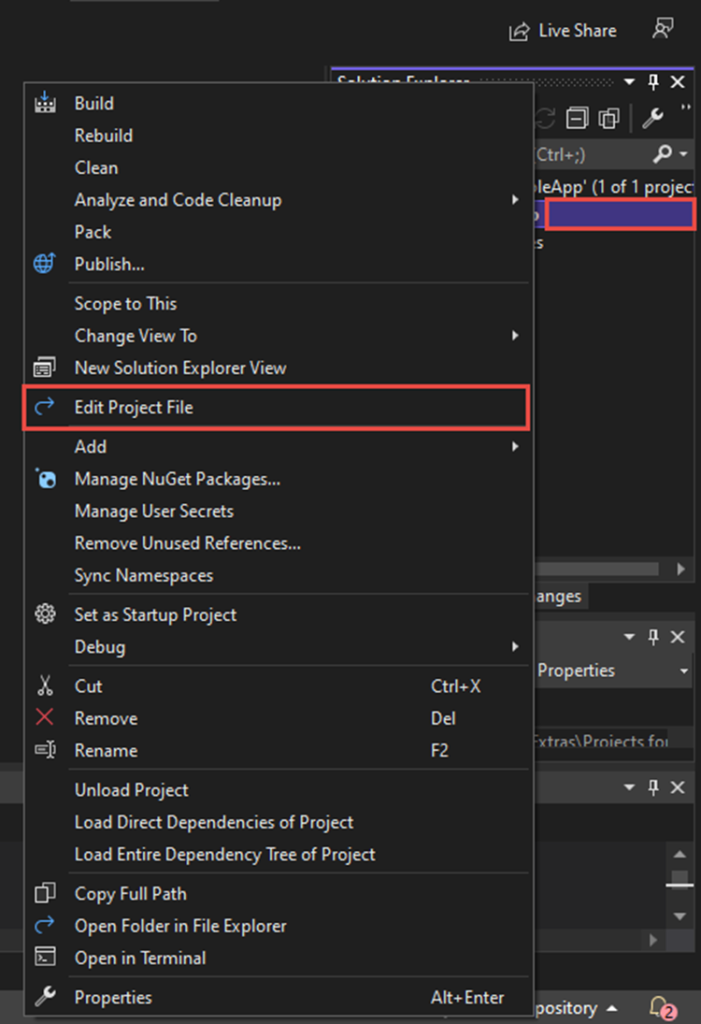
Then project file will be opened, and you can simply disable Nullable as illustrated below.
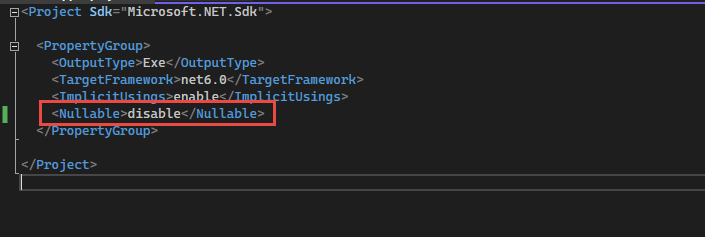
Inside the PropertyGroup change Nullable to disable as shown below or remove that line.
<Nullable>disable</Nullable>
Method 2: Giving default value
You can assign it in different ways:
a default value
reasonable default value as a string.Empty or
“”
as illustrated in the below code.
public class Person {
public string Name = "Default Value";
public string Sex = "";
public string Address = "";
public string Email = string.Empty;
public string Description {
get;
set;
} = string.Empty;
}
Method 3
Another way to handle it is to make properties nullable reference type by simply using "?" as demonstrated below.
public class Person {
public string ? Name;
public string ? Sex;
public string ? Address;
public string ? Email;
public string ? Description {
get;
set;
}
}
Overall, in .NET 6 we should define the variable, properties, or field explicitly as either nullable or non-nullable with a reference type.
Summary
In this article, I have created a console application in .NET 6 and demonstrated issues with fields or properties if we code as in .NET 5 or lower. Additionally, the article has provided the different methods to handle such situations in .NET 6. Hence, these are the three ways to handle the nullable, non-nullable field and properties in .NET 6. I hope you have got an idea about it.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
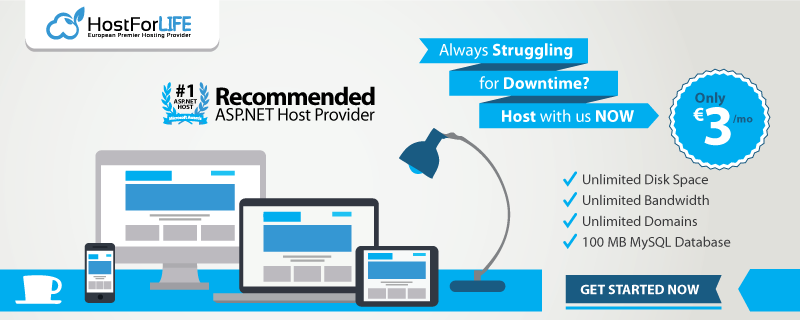