
August 30, 2021 07:01 by
Peter
In today’s article, we will take a look at the new PriorityQueue collection introduced with .NET 6. This is an advanced version of the existing queue collection. It allows us to add the priority of an item when the item is added to the queue. We will be using the Visual Studio 2022 preview version for this article. So, let us begin.
Creating our console application in Visual Studio 2022
Let us create a console application in Visual Studio 2022. I am using the community preview edition.
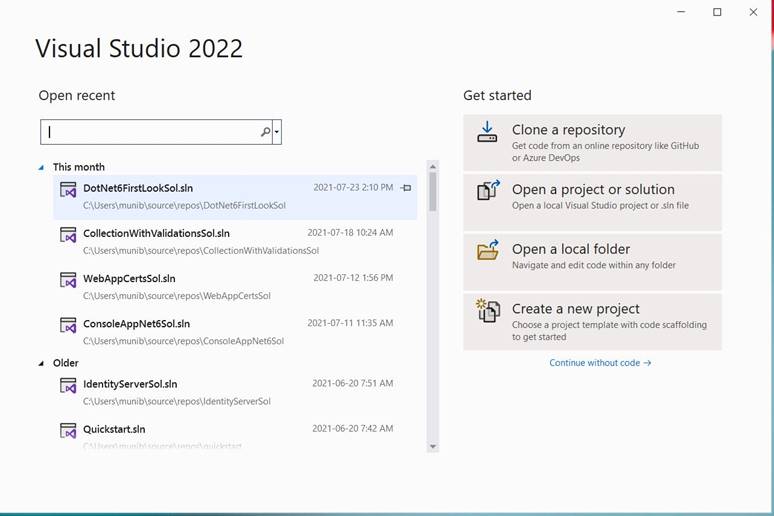
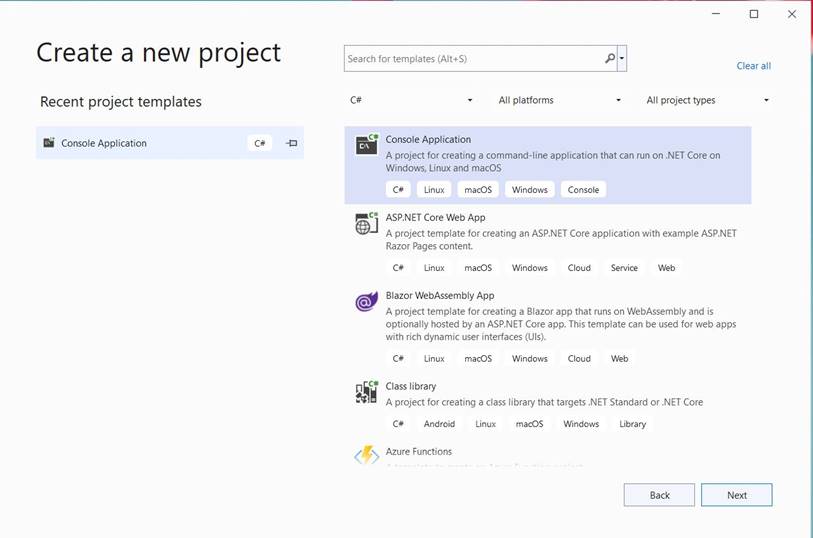
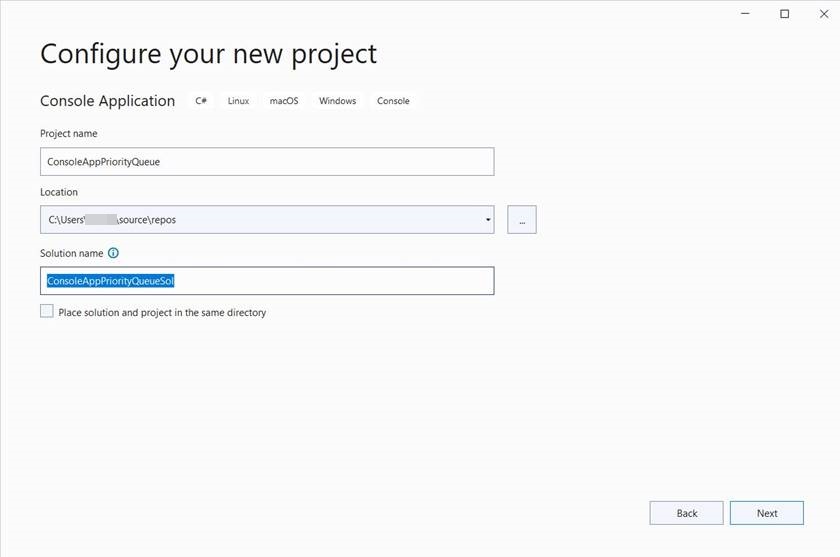
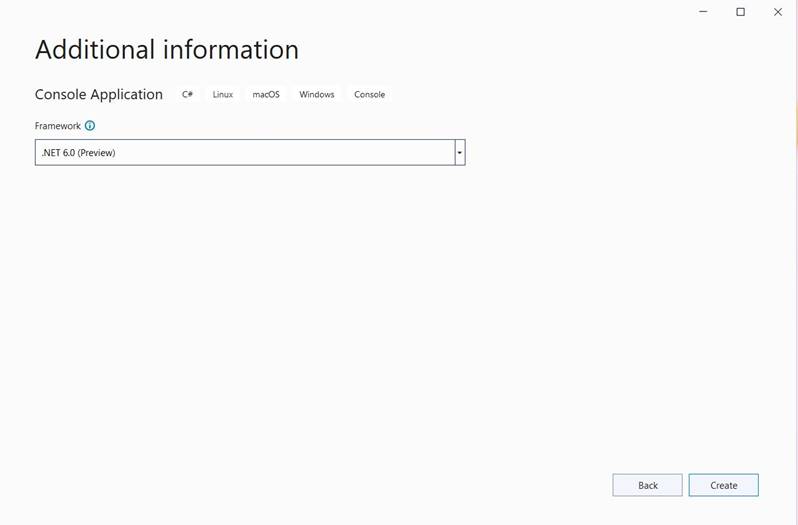
We now see the below,
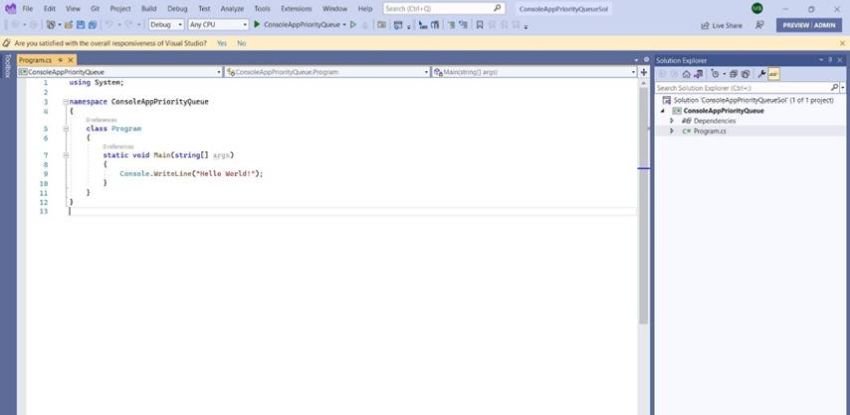
Add the below code in the “Program.cs” file.
using System;
using System.Collections.Generic;
// Old Queue implementation
Console.WriteLine("Old Queue implementation");
var numbers = new Queue < string > ();
numbers.Enqueue("one");
numbers.Enqueue("two");
numbers.Enqueue("three");
numbers.Enqueue("four");
var total = numbers.Count;
for (var i = 0; i < total; i++) {
var number = numbers.Dequeue();
Console.WriteLine(number);
}
// New C#10 PriorityQueue
Console.WriteLine("New C#10 PriorityQueue implementation");
var newNumbers = new PriorityQueue < string,
int > ();
newNumbers.Enqueue("one", 3);
newNumbers.Enqueue("two", 4);
newNumbers.Enqueue("three", 2);
newNumbers.Enqueue("four", 1);
var newTotal = newNumbers.Count;
for (var i = 0; i < newTotal; i++) {
var number = newNumbers.Dequeue();
Console.WriteLine(number);
}
Console.ReadKey();
Looking at the above code we see that we first create a simple queue collection and enqueue four strings to it. When we dequeue the elements of the queue collection, we get them back in the same order in which they were added. This is the default working of the queue.
However, in the second part of the code, we are using the new PriorityQueue collection in which we can specify the order in which we would want to dequeue the elements. Hence, we are giving the members of the queue a priority.
When we run the code, we will see the below,
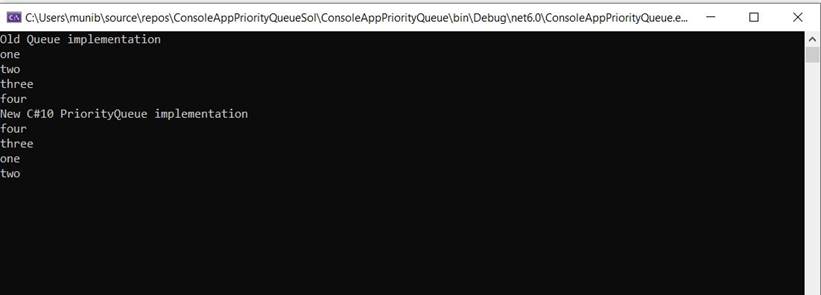
In this article, we took a look at the new PriorityQueue collection introduced with .NET 6. This can be very useful for creating a collection in which certain members need to be dequeued and processed before others irrespective of when they were added to the queue. Happy coding!
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
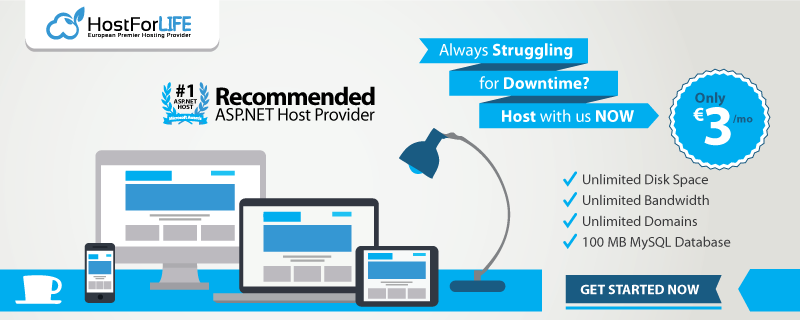