
May 25, 2015 07:48 by
Peter
Today I'll show you the way to make a search in GridView in ASP.NET using C#. You'll do it normally getting a ASP command button and run a query to get the value in a GridView. It'll refresh the page every time. You'll stop this by adding an UpdatePanel but the time taking will be more or less same. however here I'll use quickseacrch.js to search within a GridView. To do this produce a new project and add a new web form in your Visual Studio. In the aspx file write the following code to proceed.
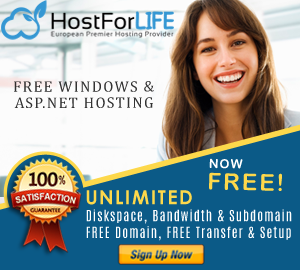
<form id="form1" runat="server">
<asp:gridview autogeneratecolumns="False" headerstyle-backcolor="#3AC0F2" headerstyle-forecolor="White" id="GridView1" ondatabound="OnDataBound" runat="server">
<columns>
<asp:templatefield headertext="Name">
<itemtemplate>
<asp:label id="Label1" runat="server" text="<%# Eval("Name") %>"></asp:label>
</itemtemplate>
</asp:templatefield>
</columns>
</asp:gridview>
<script type="text/javascript">
$(function () {
$('.search_textbox').each(function (i) {
$(this).quicksearch("[id*=GridView1] tr:not(:has(th))", {
'testQuery': function (query, txt, row) {
return $(row).children(":eq(" + i + ")").text().toLowerCase().indexOf(query[0].toLowerCase()) != -1;
}
});
});
});
</script>
</form>
Now, in the head section add a script tag to do this js function:
<script type="text/javascript">
$(function () {
$('.search_textbox').each(function (i) {
$(this).quicksearch("[id*=GridView1] tr:not(:has(th))", {
'testQuery': function (query, txt, row) {
return $(row).children(":eq(" + i + ")").text().toLowerCase().indexOf(query[0].toLowerCase()) != -1;
}
});
});
});
</script>
In the CS page write the following code:
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
DataTable dt = new DataTable();
dt.Columns.AddRange(new DataColumn[1] {new DataColumn("Name", typeof(string))});
dt.Rows.Add("Peter");
dt.Rows.Add("Scott");
dt.Rows.Add("Rebecca");
dt.Rows.Add("Suzan");
dt.Rows.Add("Tom");
GridView1.DataSource = dt;
GridView1.DataBind();
}
}
protected void OnDataBound(object sender, EventArgs e)
{
GridViewRow row = new GridViewRow(0, 0, DataControlRowType.Header, DataControlRowState.Normal);
for (int i = 0; i < GridView1.Columns.Count; i++)
{
TableHeaderCell cell = new TableHeaderCell();
TextBox txtSearch = new TextBox();
txtSearch.Attributes["placeholder"] = GridView1.Columns[i].HeaderText;
txtSearch.CssClass = "search_textbox";
cell.Controls.Add(txtSearch);
row.Controls.Add(cell);
}
GridView1.HeaderRow.Parent.Controls.AddAt(1, row);
}
Finally, run the code. Hope it works!
Free ASP.NET 5 Hosting
Try our Free ASP.NET 5 Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc.
