Normally, you used to have some data in appSettings section of web.config and read it when required. That is in string form, but there are lot more than this and you can update the data in web.config programmatically as well. You can store some object of custom type in web.config as well, which you normally don’t do it. But this can be very useful in several scenarios.
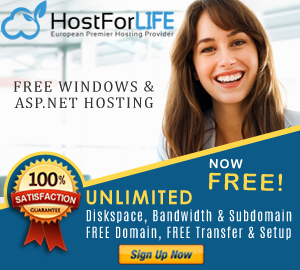
Have anyone tried to update some value or add some value in web.config? In this post, I'm going to tell you about web.config and how you can store the costum objects in web.config.
First, this is very common to have some constant data at appSettings section of web.config and read it whenever required. This is the way how to read data at appSettings:
//The data is stored in web.config as
<appSettings>
<add key="WelcomeMessage" value="Hello All, Welcome to my Website." />
</appSettings>
// To read it
string message = ConfigurationManager.AppSettings["WelcomeMessage"];
If you want to update some data of appSettings programatically, you can do like this.
//Update header at config
Configuration config = WebConfigurationManager.OpenWebConfiguration(Request.ApplicationPath);
config.AppSettings.Settings["WelcomeMessage"].Value = "Hello All, Welcome to my updated site.";
config.Save();
If you want to add some data in appSettings, you can add some app.config data as below:
//Update header at config
Configuration config = WebConfigurationManager.OpenWebConfiguration(Request.ApplicationPath);
config.AppSettings.Settings.Add("ErrorMessage", "An error has been occured during processing this request.");
config.Save();
The above code is adding one new key value pair in web.config file. Now this can be read anywhere in the application.
Now, the question is, Can we store some custom data at config? The answer is YES! We can store some object. Let’s see how:
In this example, I have saved an object of my custom class NewError in web.config file. And also updating it whenever required.
To do this, Follow the below steps.
Step 1
Create a Class that inherit From ConfigurationSection (It is available under namespace System.Configuration ). Every property must have an attribute ConfigurationProperty, having attribute name and some more parameters. This name is directly mapped to web.config. Let’s see the NewError class:
public class NewError:ConfigurationSection
{
[ConfigurationProperty ("Id",IsRequired = true)]
public string ErrorId {
get { return (string)this["Id"]; }
set { this["Id"] = value; }
}
[ConfigurationProperty("Message", IsRequired = false)]
public string Message {
get { return (string)this["Message"]; }
set { this["Message"] = value; }
}
[ConfigurationProperty("RedirectURL", IsRequired = false)]
public string RedirectionPage
{
get { return (string)this["RedirectURL"]; }
set { this["RedirectURL"] = value; }
}
[ConfigurationProperty("MailId", IsRequired = false)]
public string EmailId
{
get { return (string)this["MailId"]; }
set { this["MailId"] = value; }
}
[ConfigurationProperty("DateAdded", IsRequired = false)]
public DateTime DateAdded
{
get { return (DateTime)this["DateAdded"]; }
set { this["DateAdded"] = value; }
}
}
You can see every property has attribute ConfigurationProperty with some value. As you can see the property ErrorId has attribute:
[ConfigurationProperty ("Id",IsRequired = true)]
it means ErrorId will be saved as Id in web.config file and it is required value. There are more elements in this attribute that you can set based on your requirement.
Now if you’ll see the property closely, it is bit different.
public string ErrorId {
get { return (string)this["Id"]; }
set { this["Id"] = value; }
}
Here the value is saved as the key “id”, that is mapped with web.config file.
Step 2
Now you are required to add/register a section in the section group to tell the web.config that you are going to have this kind of data. This must be in and will be as:
<section name="errorList" type="NewError" allowLocation="true"
allowDefinition="Everywhere"/>
Step 3
Now one can add that object in your config file directly as:
<errorList Id="1" Message="ErrorMessage" RedirectURL="www.google.com" MailId="[email protected]" ></errorList>
<errorList Id="1" Message="ErrorMessage" RedirectURL="www.google.com" MailId="[email protected]" ></errorList>
Step 4
And to read it at your page. Read it as follows:
NewError objNewError = (NewError)ConfigurationManager.GetSection("errorList");
And also a new object can be saved programmatically as
NewError objNewError = new NewError()
{
RedirectionPage="www.rediff.com",
Message = "New Message",
ErrorId="0",
DateAdded= DateTime.Now.Date
};
Configuration config =
WebConfigurationManager.OpenWebConfiguration(Request.ApplicationPath);
config.Sections.Add("errorList", objNewError);
config.Save();
Even one can add a custom group and have some custom elements in in this section. ASP.NET provides very powerfull APIs to read/edit the web.config file easily.
Free ASP.NET Hosting
Try our Free ASP.NET Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc. You will not be charged a cent for trying our service for the next 3 days. Once your trial period is complete, you decide whether you'd like to continue.
