
February 5, 2024 07:05 by
Peter
I'll go over how to construct a.NET MAUI Popup page with Visual Studio 2022 in this tutorial. Operating systems offer a mechanism to display a message and request a response from the user. These alerts are usually restricted in terms of the content a developer can provide as well as the layout and appearance. Popups are a very common way of presenting information to a user that relates to their current task.
Important
- An exception will be raised when attempting to display your Popup if the code underlying the file is not generated concurrently with the call to InitializeComponent.
- Only a Page or an implementation that derives from a Page may display a Popup.
Take note
- It will finish and return to the calling thread before the operating system dismisses the Popup from the screen since Close() is a fire-and-forget procedure. Use CloseAsync() in its place if you need to stop your code from running until the operating system has removed the Popup from the screen.
- The ResultWhenUserTapsOutsideOfPopup property allows you to modify the value that is returned in order to handle tapping outside of a Popup while also waiting for the outcome.
- Make sure to specify the ApplyToDerivedTypes property on the Style definition when establishing a style that targets Popup if you want it to apply to custom popups, such as the SimplePopup example.
Step 1: Open Visual Studio 2022, create a new project, and choose the app option under the multiplatform section on the left side panel. Next, Select the.NET MAUI App with C# option and press the continue button.
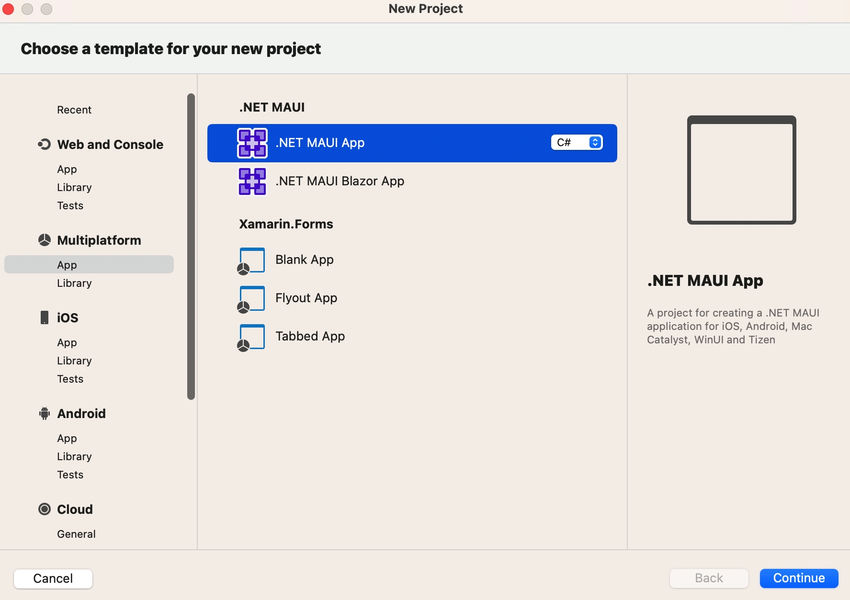
Step 2: You must choose the.Net framework version 6.0 and press the proceed button on the following screen.
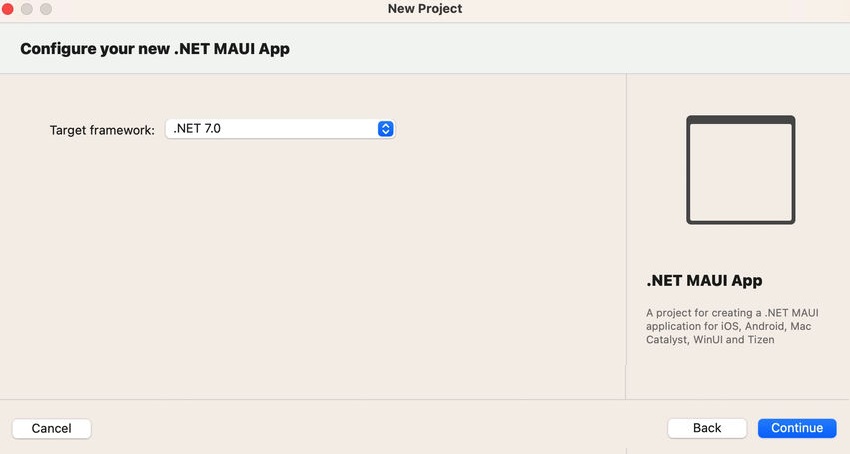
Step 3: Click the Create button after entering your location, the name of your project, and the name of your solution on the following screen.
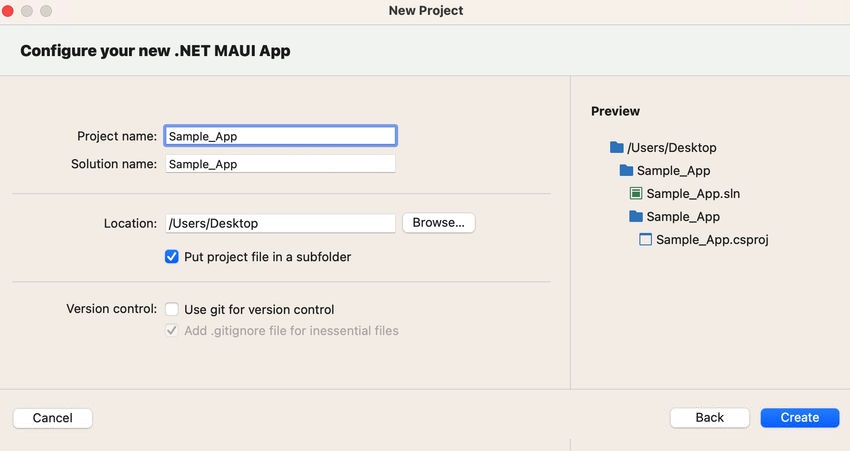
Step 4: The NuGet Package CommunityToolkit needs to be downloaded.The MauiProgram.cs file needs to be configured for Maui and CommunityToolkit.
The Community Toolkit can be downloaded using NuGet Package Manager.
dotnet add package CommunityToolkit.Maui --version 7.0.1
2. Configure CommunityToolKit in MauiProgram.cs.
using Microsoft.Extensions.Logging;
using CommunityToolkit.Maui;
namespace Popup
{
public static class MauiProgram
{
public static MauiApp CreateMauiApp()
{
var builder = MauiApp.CreateBuilder();
builder
.UseMauiApp<App>()
.UseMauiCommunityToolkit()
.ConfigureFonts(fonts =>
{
fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular");
fonts.AddFont("OpenSans-Semibold.ttf", "OpenSansSemibold");
});
#if DEBUG
builder.Logging.AddDebug();
#endif
return builder.Build();
}
}
}
Step 5. The next step is to create the new content page and define the Community Popup view In order to use the toolkit in XAML the following xmlns need to be added to your page or view.
xmlns:toolkit="http://schemas.microsoft.com/dotnet/2022/maui/toolkit"
Therefore, the following
<?xml version="1.0" encoding="utf-8" ?>
<mct:Popup xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:mct="clr-namespace:CommunityToolkit.Maui.Views;assembly=CommunityToolkit.Maui"
x:Class="Popup.MainPage"
CanBeDismissedByTappingOutsideOfPopup="False"
Size="300, 300">
<Border VerticalOptions="CenterAndExpand"
HorizontalOptions="CenterAndExpand"
Stroke="White"
StrokeThickness="1"
StrokeShape="RoundRectangle 10">
<VerticalStackLayout VerticalOptions="CenterAndExpand" Spacing="20">
<Label Text="Welcome to .NET MAUI!"
VerticalOptions="Center"
HorizontalOptions="Center" />
<Button Text="Close" Clicked="Button_Clicked" />
</VerticalStackLayout>
</Border>
</mct:Popup>
Step 6. The next step is to call the Popup from Main Page event or initialization and A Popup can only be displayed from a Page or an implementation inheriting from Page.
using CommunityToolkit.Maui.Views;
namespace Popup
{
public partial class FirstPage : ContentPage
{
public FirstPage()
{
InitializeComponent();
}
private void OnCounterClicked(object sender, EventArgs e)
{
this.ShowPopup(new MainPage());
}
}
}
Output screen
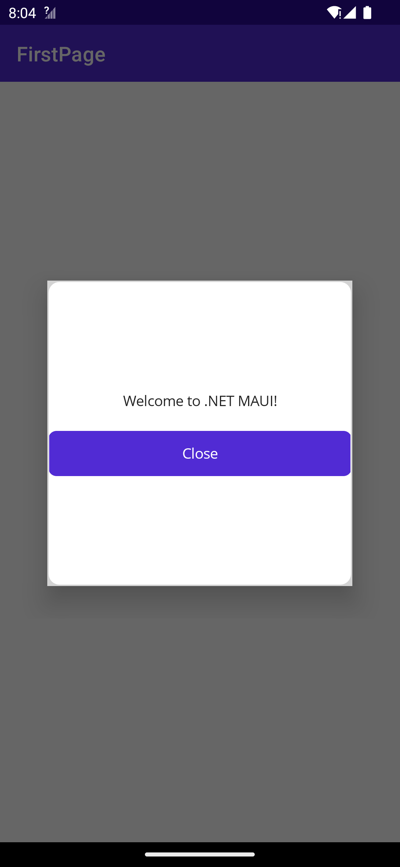
With any luck, this post has provided you with enough knowledge to use viewmodel to design an MAUI collection view and execute the application on both iOS and Android. Please feel free to leave a comment if you would like me to go into further detail on anything I've covered in this post.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
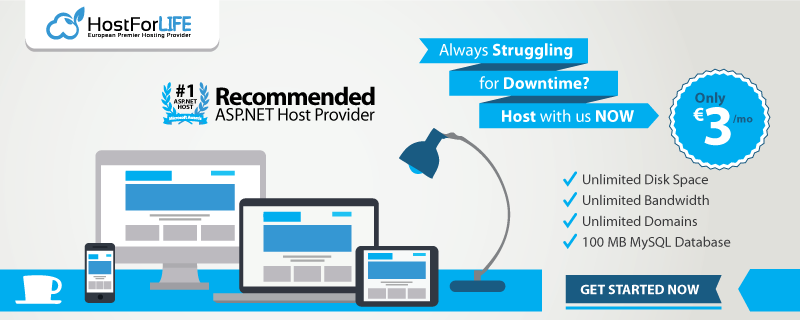