Program.cs is where the application starts. Program.cs file will work the same as Program.cs file in traditional console application of .NET Framework. Program.cs fille is the entry point of application and will be responsible to register Startup.cs fill, IISIntegration and Create a host using an instance of IWebHostBuilder, the Main method.
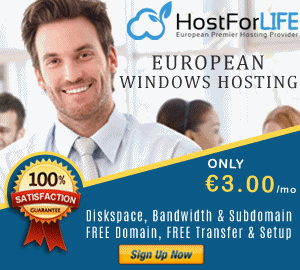
Global.asax is no longer in ASP.NET Core application. Startup.cs file is a replacement of Global.asax file in ASP.NET Core.
Startup.cs file is entry point, and it will be called after Program.cs file is executed at application level. It handles the request pipeline. Startup class triggers the second the application launches.
Description
What is Program.cs ?
Program.cs is where the application starts. Program.cs class file is entry point of our application and creates an instance of IWebHost which hosts a web application.
public class Program {
public static void Main(string[] args) {
BuildWebHost(args).Run();
}
public static IWebHost BuildWebHost(string[] args) => WebHost.CreateDefaultBuilder(args).UseStartup < startup > ().Build();
}
WebHost is used to create instance of IWebHost and IWebHostBuilder and IWebHostBuilder which are pre-configured defaults. The CreateDefaultBuilder() method creates a new instance of WebHostBuilder. UseStartup<startup>() method specifies the Startup class to be used by the web host. We can also specify our custom class in place of startup.
Build() method returns an instance of IWebHost and Run() starts web application until it stops.
Program.cs in ASP.NET Core makes it easy for us to setup a web host.
public static IWebHostBuilder CreateDefaultBuilder(string[] args) {
var builder = new WebHostBuilder().UseKestrel().UseContentRoot(Directory.GetCurrentDirectory()).ConfigureAppConfiguration((hostingContext, config) => {
/* setup config */ }).ConfigureLogging((hostingContext, logging) => {
/* setup logging */ }).UseIISIntegration()
return builder;
}
the UseKestrel() specify method is an extension, which specifies Kestrel as an internal web server. The Kestrel is an open-source, cross-platform web server for ASP.NET Core. Application is running with Asp.Net Core Module and it is necessary to enable IIS Integration (UseIISIntegration()), which configures the application base address and port.
It also configures UseIISIntegration(), UseContentRoot(), UseEnvironment("Development"), UseStartup<startup>() and other configurations are also available, like Appsetting.json and Environment Variable. UseContentRoot is used to denote current directory path.
We can also register logging and set minimium loglevel as shown below. It also overrides loglevel configured in appsetting.json file.
.ConfigureLogging(logging => { logging.SetMinimumLevel(LogLevel.Warning); })
In the same way we can also control body size of our request and response by enabling in program.cs file as below.
.ConfigureKestrel((context, options) => { options.Limits.MaxRequestBodySize = 20000000; });
ASP.net Core is cross-platform and open-source and also it is compatible to host into any server rather than IIS, external web server such as IIS, Apache, Nginx etc.
What is Startup file?
Now the question is, is startup.cs file is mandatory or not? Yes, startup.cs is mandatory, it can be decorated with any access modifier like public, private, internal. Multiple Startup classes are allowed in a single application. ASP.NET Core will select the appropriate class based on its enviroment.
If a class Startup{EnvironmentName} exists, that class will be called for that EnvironmentName or Environment Specific startup file will be executed to avoid frequent changes of code/setting/configuration based on environment.
ASP.NET Core supports multiple environment variables like Development, Production and Staging. It reads the environment variable ASPNETCORE_ENVIRONMENT at application startup and store value into Hosting Environment interface.
Should we need to define class name with startup.cs? No, it is not necessary, that class name should be Startup.
We can define two methods in startup file like ConfigureServices and Configure along with constructor.
Startup file example
public class Startup {
// Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services) {
...
}
// Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app) {
...
}
}
ConfigureServices Method
This is an optional method in Startup class which is used to configure services for application. When any request comes to the application, he ConfigureService method will be called first.
ConfigureServices method includes IServiceCollection parameter to register services. This method must be declared with a public access modifier, so that environment will be able to read the content from metadata.
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
}
Configure Method
The Configure method is used to specify how the application will respond in each HTTP request. This method is mostly used for registering middleware in HTTP pipeline. This method method accept IApplicationBuilder parameter along with some other services like IHostingEnvironment and ILoggerFactory. Once we add some service in ConfigureService method, it will be available to Configure method to be used.
public void Configure(IApplicationBuilder app)
{
app.UseMvc();
}
Above example shows how to enable MVC feature in our framework. We need to register UseMvc() into Configure and also need to register service AddMvc() into ConfigureServices. UseMvc is know as middlware. Middleware is new concept introduce with Asp.net Core, thereaew many inbuilt middleware available, and some are as below.
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
UseCookiePolicy();
UseSession();
Middleware can be configure in http pipeline using Use, Run, and Map.
Run Extension
The nature of Run extension is to short circuit the HTTP pipeline immediately. It is a shorthand way of adding middleware to the pipeline that does not call any other middleware which is next to it and immediately returns HTTP response.
Use Extension
It will transfer next invoker, so that HTTP request will be transferred to the next middleware after execution of current use if the next invoker is present.
Map Extension
Map simply accepts a path and a function that configures a separate middleware pipeline.
app.Map("/MyDelegate", MyDelegate);
To get more detailed information about middleware Click here
ASP.net core has built-in support for Dependency Injection. We can configure services to DI container using this method. Following ways are to configure method in startup class.
AddTransient
Transient objects are always different; a new instance is provided to every controller and every service.
Scoped
Scoped objects are the same within a request, but different across different requests.
Singleton
Singleton objects are the same for every object and every request.
Can we remove startup.cs and merge entire class with Program.cs ?
Answer is : Yes we can merge entire class in to single file.
Here we have gone through basic understanding of how program.cs and startup.cs file is important for our Asp.net Core application and how both can be configured, we have also had a little walk though Environment Variable and middleware and how to configure middleware and dependency injection. Additionally we have seen how UseIIsintegration() and UseKestrel() can be configured.
HostForLIFE ASP.NET 3.1.9 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
